Just add with_labels=True
to your code.
import matplotlib.pyplot as plt
import networkx as nx
socialNetworl = nx.Graph()
socialNetworl.add_nodes_from([1,2,3,4,5,6])
socialNetworl.add_edges_from([(1,2),(1,3),(2,3),(2,5),(2,6)])
nx.draw(socialNetworl, node_size = 800, node_color="cyan", with_labels = True)
plt.show()
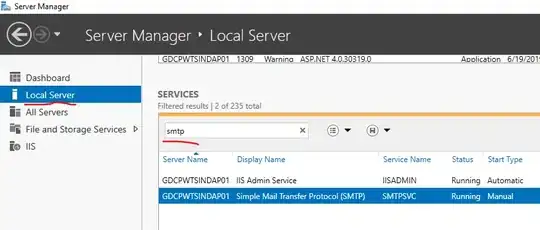
If you want to change the labels, create a dict with the labels and pass labels=theLabelDict
into nx.draw
:
import matplotlib.pyplot as plt
import networkx as nx
socialNetworl = nx.Graph()
socialNetworl.add_nodes_from([1,2,3,4,5,6])
socialNetworl.add_edges_from([(1,2),(1,3),(2,3),(2,5),(2,6)])
labels = {1:'King Arthur', 2:'Lancelot', 3:'shopkeeper', 4:'dead parrot', 5:'Brian', 6:'Sir Robin'}
nx.draw(socialNetworl, node_size = 800, node_color="cyan", labels=labels, with_labels = True)
plt.show()
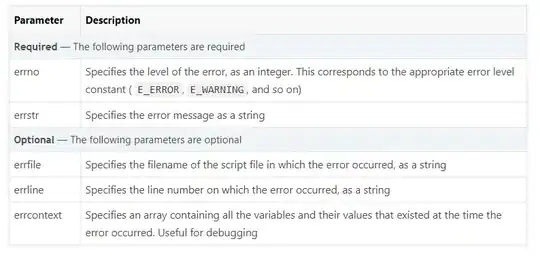