I want someone who knows what he is doing, not just copying random
answers from internet
Well, you're not going to do any better than a nine-path:
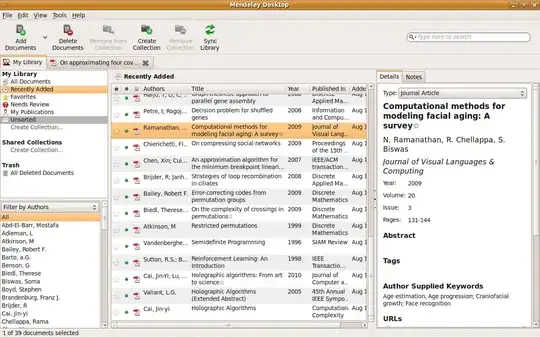
The 9-patch above has a 0.5dp (base xhdpi)
yellow border for illustration purposes. The top and left guards(black lines) define the stretchable area.
Notice that the guard line on the right runs full, end to end. This means there won't be any padding on the top and bottom. But, the guard line on the bottom starts on the leftmost px, and finishes just before the yellow border. When you set this 9patch to the listview, the bottom guard line will ensure a 0.5dp(width of the yellow border) padding to the right.
This approach does require some work though. You will need to create as many 9patch drawables as there are buckets. If you need a 0.5dp border, the width of yellow border will differ for each bucket:
xxxhdpi: 2 px
xxhdpi: 1.5 px
xhdpi: 1 px
hdpi: 0.75 px
.... and so on
More on 9patch drawables: Link
Another approach (already suggested here) is to use a View
to act as the border. In that case, the lightest arrangement (not mentioned here) is to use a FrameLayout and place the ListView and the border inside it:
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<!-- marginRight set to 0.5dp -->
<ListView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginRight="0.5dp"
android:background="#E7E7E7"
android:choiceMode="singleChoice"
android:dividerHeight="0.5dp" />
<!-- gravity set to right & background set to border color -->
<View
android:layout_width="0.5dp"
android:layout_height="match_parent"
android:layout_gravity="right"
android:background="@color/some_color"/>
</FrameLayout>
You will end up having a ViewGroup & a View as decorations in this case. 9patch would be the optimal solution. Take your pick.