To complement @arjenve's answer. To plot a Unicode character, firstly, find which font contains this character, secondly, use that font to plot characters with Matplotlib
Find a font which contains the character
According to this post, we can use fontTools package to find which font contains the character we want to plot.
from fontTools.ttLib import TTFont
import matplotlib.font_manager as mfm
def char_in_font(unicode_char, font):
for cmap in font['cmap'].tables:
if cmap.isUnicode():
if ord(unicode_char) in cmap.cmap:
return True
return False
uni_char = u"✹"
# or uni_char = u"\u2739"
font_info = [(f.fname, f.name) for f in mfm.fontManager.ttflist]
for i, font in enumerate(font_info):
if char_in_font(uni_char, TTFont(font[0])):
print(font[0], font[1])
This script will print a list of font paths and font names (all these fonts support that Unicode Character). Sample output shown below
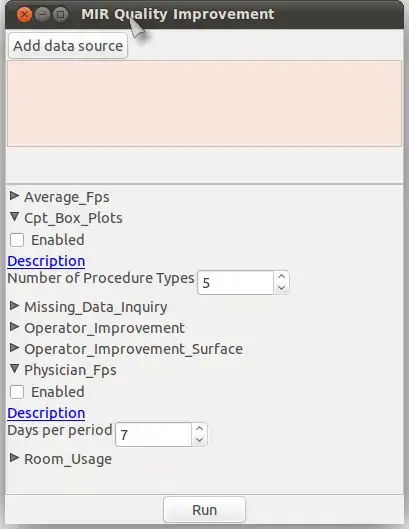
Then, we can use the following script to plot this character (see image below)
import matplotlib.pyplot as plt
import matplotlib.font_manager as mfm
font_path = '/usr/share/fonts/gnu-free/FreeSerif.ttf'
prop = mfm.FontProperties(fname=font_path)
plt.text(0.5, 0.5, s=uni_char, fontproperties=prop, fontsize=20)
plt.show()
