I don't really know how you are printing your <li>
element.
First of all, your tags inside the <li
are not valid, you should not use the "-" character inside a data
, therefore I would suggest you to change your HTML to this:
<li data-thumbnailPath="https://www.bitblokes.de/wp-content/uploads/2011/01/crunchbang-linux-logo-150x150.png"
data-url="https://www.bitblokes.de/wp-content/uploads/2011/01/crunchbang-linux-logo-150x150.png"
data-thumbnailOverlayColor="">
<div>
<p class="gallery1DecHeader">First description</p>
</div>
</li>
Note: the image is a PLACEHOLDER in this html, change it to what you have in your own page.
That said, just to give you a tip, here is a little snippet to do something that will insert into the data-thumbnailOverlayColor
the HEX of the RGB data of the image provided inside data-thumbnailPath
:
Javascript:
First, we have to write a function to convert the RGB result to a valid HEX code.. And we're going to steal that here: RGB to Hex and Hex to RGB
function componentToHex(c) {
var hex = c.toString(16);
return hex.length == 1 ? "0" + hex : hex;
}
function rgbToHex(r, g, b) {
return "#" + componentToHex(r) + componentToHex(g) + componentToHex(b);
}
Second, we're going to loop through EACH <li>
element of the DOM and we will create an image object from the data-thumbnailPath. From that image object, we will temporarely create an instance of ColorFinder, retrieve the Most prominent color and PARSE it inside the data-thumbnailOverlayColor :
var liIterator = document.getElementsByTagName("li");
for (var i = 0; i < liIterator.length; i++) {
var img = new Image();
img.src = liIterator[i].dataset.thumbnailPath;
var rgb = new ColorFinder().getMostProminentColor(img);
liIterator[i].setAttribute("data-thumbnailOverlayColor", rgbToHex(rgb.r, rgb.g, rgb.b));
}
The output is the following [visual]:
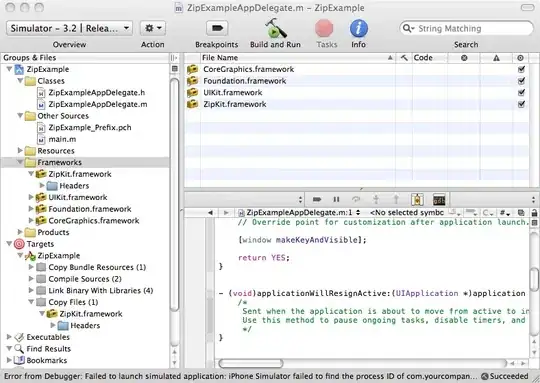
And the HTML output is this:
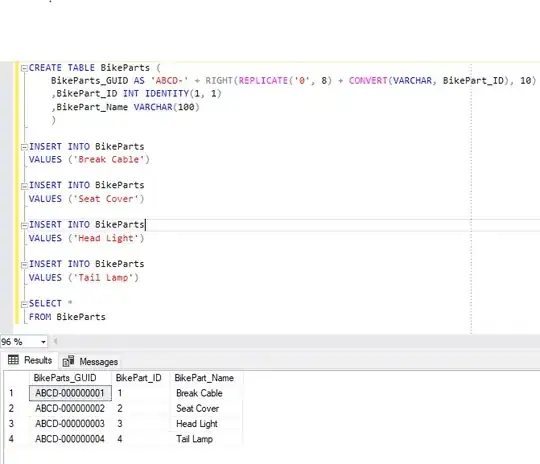
The working fiddle can be found here:
http://jsfiddle.net/82bo2wtx/
EDIT:
I'm wrong about the tag elements, "-" is accepted, but the above example works too.
In order to give you less work to do, here is the fiddle working for YOUR specific case: you just have to copy the following in a script and include that in your document or, even easier, at the very bottom of your document (just slightly before the </body>
, write this:
<script type="javascript">
var liIterator = document.getElementsByTagName("li");
for (var i = 0; i < liIterator.length; i++) {
var img = new Image();
img.src = liIterator[i].dataset["thumbnail-path"];
var rgb = new ColorFinder().getMostProminentColor(img);
liIterator[i].setAttribute("data-thumbnail-overlay-color", rgbToHex(rgb.r, rgb.g, rgb.b));
}
function componentToHex(c) {
var hex = c.toString(16);
return hex.length == 1 ? "0" + hex : hex;
}
function rgbToHex(r, g, b) {
return "#" + componentToHex(r) + componentToHex(g) + componentToHex(b);
}
</script>
http://jsfiddle.net/82bo2wtx/1/
** ASSUMING YOU HAVE ALREADY INCLUDED THE COLORFINDER LIBRARY **