I think I may understand what you want. to start I'd like to keep it simple.
I'll take it as far as you want.
Start with a simple array.
[0] => 0
[1] => 1
[2] => 2
[3] => 3
[4] => 4
[5] => 5
[6] => 6
then use it to create some divs, each div with a checkbox and text input.
echo <<<EOT
<div id="d$key" class="row">
<input type="text" id="t$key" name="txt$key" value="Text #$key" />
<input type="checkbox" id="c$key" name="chk$key" value="$key" onclick="ck($key)"/>Checkbox #$key
</div><br/>
EOT;
}
The above code produces the HTML below:
<div id="d0" class="row">
<input type="text" id="t0" name="txt0" value="Text #0" />
<input type="checkbox" id="c0" name="chk0" value="0" onclick="ck(0)"/>Checkbox #0
</div><br/><div id="d1" class="row">
<input type="text" id="t1" name="txt1" value="Text #1" />
<input type="checkbox" id="c1" name="chk1" value="1" onclick="ck(1)"/>Checkbox #1
</div><br/><div id="d2" class="row">
<input type="text" id="t2" name="txt2" value="Text #2" />
<input type="checkbox" id="c2" name="chk2" value="2" onclick="ck(2)"/>Checkbox #2
</div><br/><div id="d3" class="row">
<input type="text" id="t3" name="txt3" value="Text #3" />
<input type="checkbox" id="c3" name="chk3" value="3" onclick="ck(3)"/>Checkbox #3
</div><br/><div id="d4" class="row">
<input type="text" id="t4" name="txt4" value="Text #4" />
<input type="checkbox" id="c4" name="chk4" value="4" onclick="ck(4)"/>Checkbox #4
</div><br/><div id="d5" class="row">
<input type="text" id="t5" name="txt5" value="Text #5" />
<input type="checkbox" id="c5" name="chk5" value="5" onclick="ck(5)"/>Checkbox #5
</div><br/><div id="d6" class="row">
<input type="text" id="t6" name="txt6" value="Text #6" />
<input type="checkbox" id="c6" name="chk6" value="6" onclick="ck(6)"/>Checkbox #6
</div>
Which looks like:
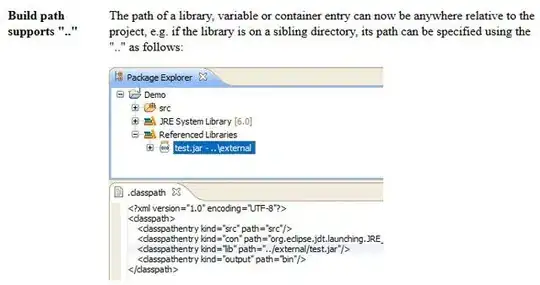
each check box has an onclick event the calls the function ck() and passes the number associated with the check box, div, and text.
Each row with text and check box all have related id.
Check box id="c#"
Text id = "t#"
div id="d#"
Where # is the row number.
To show it can do something I have the function ck() changing the background color of the text and div in it own row.
function ck(id){
var div = document.getElementById('d' + id);
var checked = document.getElementById('c' + id).checked;
div.style.backgroundColor = color[checked];
var txt = document.getElementById('t' + id);
txt.style.backgroundColor = color[checked];
}
the background color is based on whether the check box is check or un-checked.
I create a global array because I avoid using if else do to its inefficiencies.
var color = new Array;
color[true] = '#ff0';
color[false] = '#f00';
The first thing the ck(0 function does is get the checkbox checked property.
var checked = document.getElementById('c' + id).checked;
Then retrieve the text and div elements
var div = document.getElementById('d' + id);
var txt = document.getElementById('t' + id);
Then set their colors based on the check box using the var checked
above.
div.style.backgroundColor = color[checked];
txt.style.backgroundColor = color[checked];
This is how it looks when the check boxes are checked and unchecked:
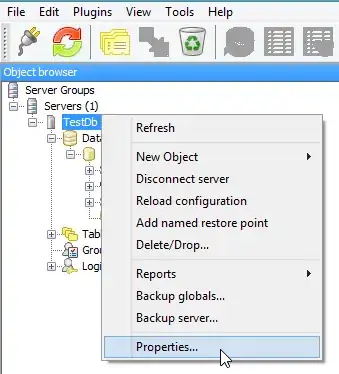
The complete source:
or here is a link to a working version: Demo of the code
This code is test and debugged.
- No HTML errors (W3C HTML Validator)
- No CSS Errors (W3C CSS Valadator)
- 100% Mobile Friendly (W3C mobileOK)
- Yahoo YSlow! 100%
- Google PageSpeed 99% (bug in their checker deducts 1%)
- Google PageSpeed Insights Mobile 100%
- Google PageSpeed Insights Destop 100%
<?php ob_start("ob_gzhandler");
header('Content-Type: text/html; charset=utf-8');
header('Connection: Keep-Alive');
header('Keep-Alive: timeout=5, max=100');
header('Cache-Control: max-age=84600');
header('Vary: Accept-Encoding');
echo <<<EOT
<!DOCTYPE html>
<html lang="en"><head><title>Testbed</title><meta name="viewport" content="width=device-width, initial-scale=1.0" />
<style type="text/css">
.row{display:inline;}
</style></head><body><div id="page">
<form action="#" method="post"><div>
EOT;
ob_flush();
for($i=0;$i<7;$i++){$divs[] = $i;}
foreach($divs as $key => $val){
echo <<<EOT
<div id="d$key" class="row">
<input type="text" id="t$key" name="txt$key" value="Text #$key" />
<input type="checkbox" id="c$key" name="chk$key" value="$key" onclick="ck($key)"/>Checkbox #$key
</div><br/>
EOT;
}
ob_flush();
echo <<<EOT
</div></form></div>
<script type="text/javascript">
//<![CDATA[
var divs = document.getElementsByTagName("div");
var color = new Array;
color[true] = '#ff0';
color[false] = '#f00';
function ck(id){
var div = document.getElementById('d' + id);
var txt = document.getElementById('t' + id);
var checked = document.getElementById('c' + id).checked;
div.style.backgroundColor = color[checked];
txt.style.backgroundColor = color[checked];
}
//]]>
</script></body></html>
EOT;
ob_end_flush();
?>