You are missing some information, so this is basically a very educated guess:
I assume you are using Autolayout for this (probably should have tagged it).
The behavior you see can be caused if you have set preferredMaxLayoutWidth
of the label to a higher value than what is actually used.
I played around a bit and can now reproduce the result.
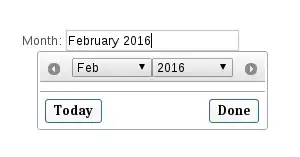
As you can see (click it), I set preferredMaxLayoutWidth
to 310 in line 13. But the actual width of the label is 299 points (line 36).
The Autolayout engine will use 310 instead of 299 to calculated how many lines are needed. The first strings fits in a line that is 310 pt. wide, the second string does not. That's why only the text in the first label is truncated.
The solution is to not set preferredMaxLayoutWidth
. This might have a performance impact or any other negative consequences though. In this case you have to figure out a way to set it to the correct value.
Here is the complete playground, in case someone wants to play with it:
// Playground - noun: a place where people can play
import UIKit
var view = UIView(frame: CGRect(x: 0, y: 0, width: 315, height: 200))
view.backgroundColor = UIColor.lightGrayColor()
func addLabel(view: UIView) -> UILabel {
let label1 = UILabel()
label1.numberOfLines = 0
label1.backgroundColor = UIColor.redColor().colorWithAlphaComponent(0.1)
label1.setTranslatesAutoresizingMaskIntoConstraints(false)
// label1.preferredMaxLayoutWidth = 310
view.addSubview(label1)
return label1
}
let label1 = addLabel(view)
let label2 = addLabel(view)
let views = ["l1" : label1, "l2" : label2]
view.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat("V:|-[l1]-8-[l2]", options: nil, metrics: nil, views: views))
view.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat("H:|-[l1]-|", options: nil, metrics: nil, views: views))
view.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat("H:|-[l2]-|", options: nil, metrics: nil, views: views))
label1.text = "How to Wear This Spring's Must-Haves"
label2.text = "The Best New Men's Fragrances For Spring 2015"
view.layoutIfNeeded()
view.frame
label1.frame
label1.preferredMaxLayoutWidth
label2.frame
label2.preferredMaxLayoutWidth