I usually use the following pattern
angular.module('yourServiceName.car.model', [])
.factory('Car', function() {
function Car(car) {
/* extend with lodash.
* Since this is a POJO, don't transform anything you dont need.
*/
_.assign(this, car, this);
}
Car.Enums = {SOME:1, ENUM:2, YOU:3, MIGHT:4, NEED:5};
Car.prototype.method = function() {
/* some model specific method.
* don't throw REST or UI things here tho.
*/
}
Car.create = {
fromJSON: function(json) {
/* only use this for JSON missing transformations, like
* 'Y'|'N' to true|false
* dateString to Date instance
*/
json.startDate = Date.parse(json.startDate); or something.
// I want to see 'Car' in my debug window, instead of 'Object'
return new Car(json);
}
}
return Car;
});
Then on your API service
angular.module('yourService.car.rest', [
'yourService.car.model'
])
.factory('carApi', function(baseURL, Car) {
var path = baseURL+'/car'
var Routes = {
CREATE : path,
LIST : path+'/%s',
DETAILS: path+'/%d'
};
function getCar(id, params) {
// this should blowup if id is not a number
var url = sprintf(Routes.DETAILS, id);
return $http.get(url, params).then(function(response.data) {
// if now JSON transformations are done
// return new Car(response.data);
return Car.create.fromJSON(response.data);
});
}
function listCars(ids, params) {
ids = ids || [];
var _ids = ids.join(',');
var url = sprintf(Routes.LIST, _ids);
return $http.get(url, params).then(function(response) {
return _.map(response.data, Car.create.fromJSON);
});
}
function createCar(oCar) {
/* Hungarian notation indicates I expect an instance of 'Car'
* And not just any object
*/
$http.post(Routes.CREATE, {data: oCar});
}
return {list:listCars, get:getCar, create:createCar};
});
So finally in your controller, you would have something like
angular.module('yourProject.ui.car.list', [
'yourServiceName.car.model',
'yourServiceName.car.rest'
])
.controller('ListCarsController', function ListCarsCtrlr(carsApi, Car) {
$scope.ids = [1, 2, 3];
$scope.load = function() {
var params = {}; // anything else you need to pass
carsApi.list(ids, params).then(function(cars) {
$scope.cars = cars; // all of these are now instanceof Car
})
}
});
Your controller ends up as simple as this:
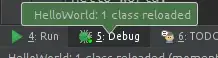
You can even take it up a nodge if you need Viewmodel
angular.module('yourProject.ui.car.list.viewmodel', [
'yourService.car.model'
])
.factory('CarItemViewmodel', function CarItemVM() {
function CarItemViewmodel(oCar) {
// do some flattening or something you can unit test
this.price = oCar.additionalAttributes.somethingDeep.price;
this.ammount = oCar.someOtherStuff[0].quantity;
};
return CarItemViewmodel;
});