You have to use sprintf("%u", $ip)
, like this:
if (sprintf("%u", $ip) <= sprintf("%u", $high_ip) && sprintf("%u", $low_ip) <= sprintf("%u", $ip))
Why? If you look into the manual: http://php.net/manual/en/function.ip2long.php
You will see it why:
Note:
Because PHP's integer type is signed, and many IP addresses will result in negative integers on 32-bit architectures, you need to use the "%u" formatter of sprintf() or printf() to get the string representation of the unsigned IP address.
EDIT:
As from the comments:
Good to know, but for what evil reason is it working properly here? prntscr.com/6i0o82 Because it might not be a 32-bit architecture? – briosheje 12 mins ago
Yes ^ there in the online IDE it works, because it's a 64bit architecture. And as mentioned above in the quote on 32-bit architectures this won't work, because it results in negative interges, so that's why you have to use %u
+ sprintf()
or printf()
.
Which architecture do I have?
You can simply do this:
echo PHP_INT_MAX;
2147483647 -> 32 bit
9223372036854775807 -> 64 bit
EDIT 2:
Also from the comments:
btw I am using xampp, but it shouldn't matter in this case? – user1721135 22 mins ago
PHP doesn't support 64 bit on windows or at least not official. You can see/read this here: http://windows.php.net/
And a quote from there:
x86_64 Builds
The x64 builds of PHP for Windows should be considered experimental, and do not yet provide 64-bit integer or large file support. Please see this post for work ongoing to improve these builds.
So you're probably using xampp on windows and there is no xampp with php 64 bit version:
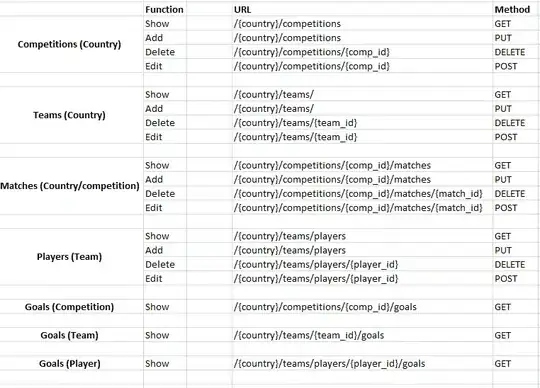
And if you are not using xampp on windows you can download xampp with 64bit php here for MAC and linux: https://www.apachefriends.org/download.html