I think that you should make situation a little bit easier before trying to detect logo - a good point to start might be applying perspective transform to make logo less distorted. Here is code which applys perspective transofrm to image:
import cv2
import numpy as np
src = np.array([[8,125], [68,30], [576,261], [510,396]], np.float32)
dst = np.array([[0,0], [100, 0], [100, 400], [0, 400]], np.float32)
perspective_transform = cv2.getPerspectiveTransform(src, dst)
img = cv2.imread('d:\\temp\\logo_lamer.png')
final_img = cv2.warpPerspective(img, perspective_transform, (100, 400))
cv2.imwrite('d:\\temp\\logo_lamer2.png', final_img)
Points are hardcode, but finding them shouldn't be very hard - i've just used left-most, top-most, right-most and bottom-most points of the bottle. Here is image with points:

and here is the result of transformation:
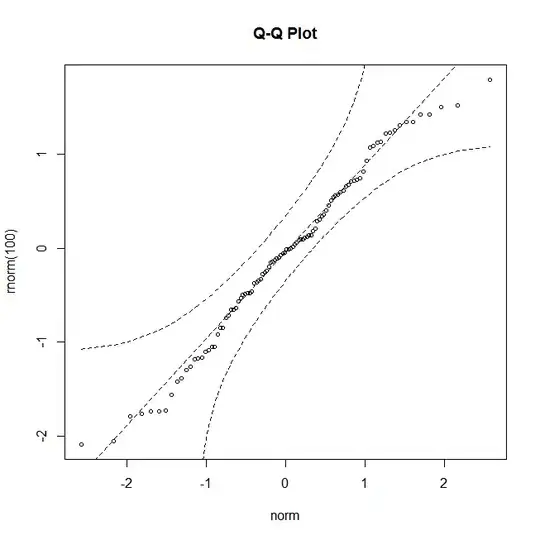
As you can see it's not perfect (letters "L" and "R" are not fully visible), but it can be imporoved using some good for object extraction. Using grabcut might be a good idea, but most likely a lot of techniques will perform well in this situation. Alternatively after finding points you can try to just make sure that they form a (rotated) rectangle, so make sure that all angles are equal (or close to) 90 degress - here is how you can do it.
Now it should be much easier to apply some technique from this qeustion (i would try scanline and sift/surf techniques, but it's just my guess). Alternatively you can try to use Generalized Hough Transfom(GHT), template matching or train you own classifier using Haar-like features, LBP(local binary patterns) or HOG.