@dfsq's solution is a nice straight forward one, but in the interest of learning :)
To try and understand regexes, I would advice looking at the flow-chart-alike visualisation that Debuggex gives you, and experiment with substitution on Regex101. (links contain the regex unaltered from your question)
I would make some small modifications:
- The
.
doesn't match new-lines, one way to do that is to match [\s\S]
in stead, which matches absolutely every character
- This:
[^\s]+\s\s*
can be untangled and optimized to \S+\s+
, but I would turn it around and make it \s*\S+
so that the ...
comes after the last word without a space in between.
Which would result in this:
((\s*\S+){40})([\s\S]*)
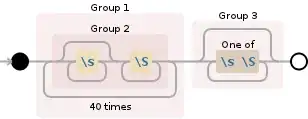
Regex101 substitution in action