If you want certain containers to work that are able to prevent duplicates of your Employee from being added you need to define how equals is calculated in Employee.
public class Employee {
//...
@Override
public boolean equals(Object obj) {
if (!(obj instanceof Person))
return false;
if (obj == this)
return true;
Person rhs = (Person) obj;
return new EqualsBuilder()
// if deriving: .appendSuper(super.equals(obj))
.append(employeeId, rhs.employeeId)
.append(firstName, rhs.firstName)
.append(lastName, rhs.lastName)
.isEquals();
}
}
If you want certain containers to work that are able to look up your Employee quickly you need to define how hashcode is calculated in Employee.
public class Employee {
//...
@Override
public int hashCode() {
int hash = 1;
hash = hash * 17 + employeeId;
hash = hash * 31 + firstName.hashCode();
hash = hash * 13 + lastName.hashCode();
return hash;
}
}
Most modern IDE's will offer a re-factoring to do this for you. Use the same fields in both so they change together.
Since you don't want duplicates you're best bet is to not use ArrayList but something that pays attention to the hash and will enforce uniqueness for you. Whenever I'm picking a container in java I look at this:
To have all of those available to you, you need to implement not only hashcode and equals but comparator as well.
Just to be clear, the point of the hash is to provide lookup speed. The point of equals is to define what objects are considered identical. The point of comparator is to provide an ordering for the objects.
If you want to include the less frequently used containers try this:
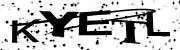