Update:
This is the result of your actual code now:
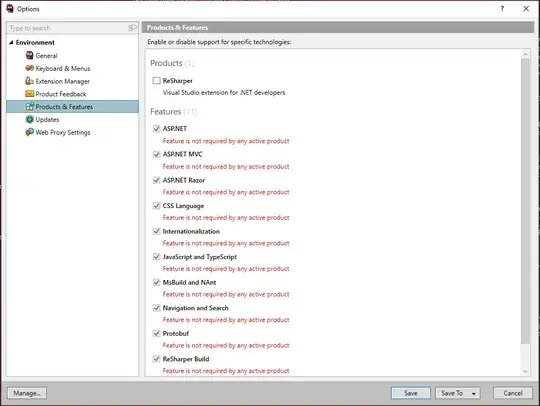
So, as you can see it is applying, but you have to know a few things:
The IMG
element embeds an image in the current document at the
location of the element's definition. The IMG
element has no content;
it is usually replaced inline by the image designated by the src
attribute, the exception being for left or right-aligned images that
are "floated" out of line.
- since you are floating the
img
tag it will start behaving like an inline-block
element
Floating an inline
or block
level element will make the element behave
like an inline-block
element (from here).
So, therefore, there is a few solutions to fix this issue:
Solution #1
(a quick fix [as you can see by adding a border
to your #container
]- I would advise to read the other solutions below)
only using you existing HTML markup and CSS:(which will make both <h1>
and <p>
indented)
- make your
img
an block element by applying display:block
(optional - to fix gap below img
tag)
- removing
margin-left
from p
and adding margin-right
to img
#container {
border:1px solid red
}
#gameImage {
width: 100px;
float: left;
height: auto;
display:block; /*new - optional*/
margin-right:10px /*new*/
}
#second {
background-color: green;
}
<div id="container">
<img src="http://boxedinfinity.com/wp-content/uploads/2013/12/Halo3.jpg" alt="Game Image" id="gameImage" />
<div id="second">
<h2>Genre:</h2>
<p>First Person Shooter</p>
</div>
</div>
Solution #2
Since you are using float
already, let's go with clearfix
.clearfix:before,
.clearfix:after {
content: " ";
display: table;
}
.clearfix:after {
clear: both;
}
#container {
background-color: green;
border:1px solid red;
}
#second {
float:left;
/* background-color: green; /*choose what block will have the background*/ }
#gameImage {
width: 100px;
float: left;
height: auto;
}
#second > p {
padding-left: 10px;
box-sizing: border-box;
<div id="container" class="clearfix">
<img src="http://boxedinfinity.com/wp-content/uploads/2013/12/Halo3.jpg" alt="Game Image" id="gameImage" />
<div id="second">
<h2>Genre:</h2>
<p>First Person Shooter</p>
</div>
</div>
Solution #3
dropping the floats
and using display:table/table-cell
#container {
display:table;
width:100%;
background-color:green;
border:1px solid red;
}
#container > div {
display:table-cell;
vertical-align:top;
}
#container > div:first-child, #container > div > img {
width:100px
}
#container > div > img {
display:block
}
#container > #second > p {
padding-left:10px
}
<div id="container">
<div>
<img src="http://boxedinfinity.com/wp-content/uploads/2013/12/Halo3.jpg" alt="Game Image" id="gameImage" />
</div>
<div id="second">
<h2>Genre:</h2>
<p>First Person Shooter</p>
</div>
</div>
Solution #4
using inline-block
#container {
width: 100%;
background-color: green;
font-size: 0;/* fix for inline-block elements gaps*/
border:1px solid red;
}
#container > div {
display: inline-block;
vertical-align: top;
font-size: 16px /* fix for inline-block elements gaps*/
}
#container > div:first-child,
#container > div > img {
width: 100px
}
#container > div > img {
display: block
}
#container > #second > p {
padding-left: 10px
}
<div id="container">
<div>
<img src="http://boxedinfinity.com/wp-content/uploads/2013/12/Halo3.jpg" alt="Game Image" id="gameImage" />
</div>
<div id="second">
<h2>Genre:</h2>
<p>First Person Shooter</p>
</div>
</div>
NOTE:
You may want to take a look at some articles regarding floats, here and here