Here is a code example for the easy part:
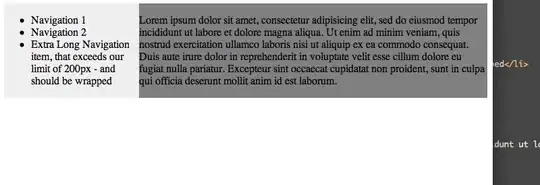
It checks for one column (0) in one row (4) and then draws the strings prepared in a list of tuples. You can change the data structure easily..
List<Tuple<SolidBrush, string>> diffStrings = new List<Tuple<SolidBrush, string>>();
private void dataGridView1_CellPainting(object sender, DataGridViewCellPaintingEventArgs e)
{
if (e.RowIndex != 4 || e.ColumnIndex != 0) { return; } // only one test-cell
float x = e.CellBounds.Left + 1;
float y = e.CellBounds.Top + 1;
e.PaintBackground(e.CellBounds,true);
foreach (Tuple<SolidBrush, string> kv in diffStrings)
{
SizeF size = e.Graphics.MeasureString(kv.Item2, Font,
e.CellBounds.Size, StringFormat.GenericTypographic);
if (x + size.Width > e.CellBounds.Left + e.CellBounds.Width)
{x = e.CellBounds.Left + 1; y += size.Height; }
e.Graphics.DrawString(kv.Item2, Font, kv.Item1, x , y);
x += size.Width;
}
e.Handled = true;
}
But where will you get the data from??
Here is how I created the test data, which are only meant to show the drawing in different colors:
SolidBrush c1 = new SolidBrush(Color.Black);
SolidBrush c2 = new SolidBrush(Color.Red);
diffStrings.Add(new Tuple<SolidBrush, string>(c1, "1234"));
diffStrings.Add(new Tuple<SolidBrush, string>(c2, "M"));
diffStrings.Add(new Tuple<SolidBrush, string>(c1, "1234"));
diffStrings.Add(new Tuple<SolidBrush, string>(c2, "ÖÄÜ"));
diffStrings.Add(new Tuple<SolidBrush, string>(c1, "1234"));
diffStrings.Add(new Tuple<SolidBrush, string>(c2, "ÖÄÜ"));
diffStrings.Add(new Tuple<SolidBrush, string>(c1, "1234"));
..
You will need to solve the problem of writing a Diff function that can fill the structure. It would help if you know restraints, like maybe that the length can't change..
You can use the Cells
' Values
and diff
them with their old values if you store these in the Cells
' Tags
. You would have to handle e.g. the CellBeginEdit
and/or CellEndEdit
event to manage the storage of the two values but the real challenge is to get the diffs, especially when there are inserted or deleted characters!
The example above aligns the text TopLeft
. Including all DataGridViewContentAlignment
options would complicate the code somewhat, but only in a limited way. The same goes for splitting after word boundaries or managing embeded line feeds.. - Writing a Diff
function however is really tough. See here for an example of how to use a ready-made one..!