You can use this trick:
<Page
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml">
<WrapPanel x:Name="mywrappanel">
<TextBox Text="Text1"/>
<TextBox Text="Text2"/>
<TextBox Text="Text3"/>
<Border Width="{Binding Path=ActualWidth, ElementName=mywrappanel}"/>
<TextBox Text="Text4"/>
<TextBox Text="Text5"/>
</WrapPanel>
</Page>
What it is doing is using a dummy element with no height (so that it is "hidden") but with a width that matches the WrapPanel
so you can be sure it can't fit on the current row, and "fills" the next one.
You can use any FrameworkElement
derived element as the dummy...just choose a lightweight one to avoid unnecessary memory usage.
You can use a binding that uses RelativeSource
if you don't want to have to name your WrapPanel
e.g.
<Page
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml">
<WrapPanel x:Name="mywrappanel">
<TextBox Text="Text1"/>
<TextBox Text="Text2"/>
<TextBox Text="Text3"/>
<Border Width="{Binding Path=ActualWidth, RelativeSource={RelativeSource Mode=FindAncestor, AncestorType={x:Type WrapPanel}}}"/>
<TextBox Text="Text4"/>
<TextBox Text="Text5"/>
</WrapPanel>
</Page>
To better visualize what it's doing...just give it a height and some colour.
<Page
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml">
<WrapPanel x:Name="mywrappanel">
<TextBox Text="Text1"/>
<TextBox Text="Text2"/>
<TextBox Text="Text3"/>
<Border Height="20" Background="Red" Width="{Binding Path=ActualWidth, ElementName=mywrappanel}"/>
<TextBox Text="Text4"/>
<TextBox Text="Text5"/>
</WrapPanel>
</Page>
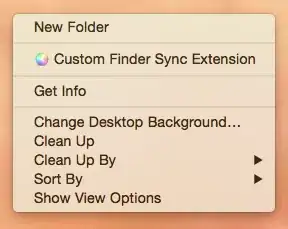
If you want your XAML to be a bit cleaner/clearer (i.e. not have the binding, and dummy Border
), then you could create your own FrameworkElement
that's designed to always match the width of an ancestor WrapPanel
.
See the NewLine
element here: