The reason this works the way it does is that you are passing strFoo
by value, not by reference. Re-assigning strFoo
inside foo
to a new string
has no effect on strMain
from main
. This is not specific to string
: all reference types in C# work in this way.
If you want to make the assignment in foo
be visible in main
, pass your string by reference:
public void Main(string[] args) {
string strMain="main";
Foo(ref strMain);
Console.WriteLine(strMain);
}
void Foo(ref string strFoo) {
strFoo="Local";
}
Note that passing a reference type by value allows the method being called to change the value of the variable that you pass, as long as that variable is mutable. This cannot be done with string
because it is immutable, but you can do it with a StringBuilder
:
public void Main(string[] args) {
StringBuilder strMain=new StringBuilder();
Foo(strMain);
Console.WriteLine(strMain);
}
void Foo(StringBuilder strFoo) {
strFoo.Append("Local");
}
Here is an illustration:
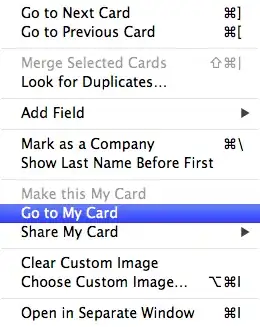