Approach
Create a lined linear gradient background for the content portion of a TextArea.
-jewelsea-lined-notepad:
linear-gradient(
from 0px 0px to 0px 11px,
repeat,
gainsboro,
gainsboro 6.25%,
cornsilk 6.25%,
cornsilk
);
Implementation Notes
The tricky part is having the lines in the linear gradient line up with the lines of text. I thought it would be a simple as specifying the gradient size as 1em (e.g. 1 measure of the font size per line), but that didn't allow the gradients to align. So I just specified the gradient in absolute pixel sizes (found by trial and error). Unfortunately, with this approach, as you change fonts or font sizes for the text area, you need to adjust the values in the gradient manually. But other than the sizing difficulty, it seems to work well.
References
Sample Output
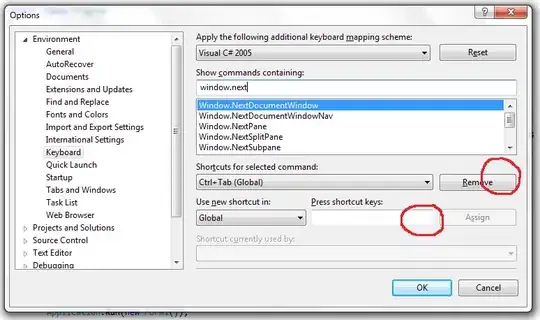
Sample Solution
Notepad.java
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.TextArea;
import javafx.scene.layout.Priority;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Notepad extends Application {
@Override
public void start(Stage stage) {
TextArea textArea = new TextArea();
Label title = new Label(
"Benjamin Franklin: Selected Quotes"
);
title.getStyleClass().add("title");
VBox layout = new VBox(
10,
title,
textArea
);
layout.setPadding(new Insets(10));
VBox.setVgrow(textArea, Priority.ALWAYS);
Scene scene = new Scene(layout);
scene.getStylesheets().add(getClass().getResource(
"notepad.css"
).toExternalForm());
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
notepad.css
.root {
-jewelsea-lined-notepad:
linear-gradient(
from 0px 0px to 0px 11px,
repeat,
gainsboro,
gainsboro 6.25%,
cornsilk 6.25%,
cornsilk
);
}
.title {
-fx-font-size: 16px;
}
.text-area {
-fx-font-family: "Comic Sans MS";
-fx-font-size: 15px;
}
.text-area .content {
-fx-background-color: -jewelsea-lined-notepad;
}
.text-area:focused .content {
-fx-background-color:
-fx-control-inner-background,
-fx-faint-focus-color,
-jewelsea-lined-notepad;
}