Make sure you set the DrawMode property of the Tab Control to OwnerDrawFixed. This property is decides whether system or developer painting the captions.
Here is code example that uses TabSizeMode = Fixed is setting the tab size:
public partial class Form1 : Form
{
const int LEADING_SPACE = 12;
const int CLOSE_SPACE = 15;
const int CLOSE_AREA = 15;
public Form1()
{
InitializeComponent();
}
private void tabControl1_DrawItem(object sender, DrawItemEventArgs e)
{
//This code will render a "x" mark at the end of the Tab caption.
e.Graphics.DrawString("x", e.Font, Brushes.Black, e.Bounds.Right - CLOSE_AREA, e.Bounds.Top + 4);
e.Graphics.DrawString(this.tabControl1.TabPages[e.Index].Text, e.Font, Brushes.Black, e.Bounds.Left + LEADING_SPACE, e.Bounds.Top + 4);
e.DrawFocusRectangle();
}
private void Form1_Load(object sender, EventArgs e)
{
// get the inital length
int tabLength = tabControl1.ItemSize.Width;
// measure the text in each tab and make adjustment to the size
for (int i = 0; i < this.tabControl1.TabPages.Count; i++)
{
TabPage currentPage = tabControl1.TabPages[i];
int currentTabLength = TextRenderer.MeasureText(currentPage.Text, tabControl1.Font).Width;
// adjust the length for what text is written
currentTabLength += LEADING_SPACE + CLOSE_SPACE + CLOSE_AREA;
if (currentTabLength > tabLength)
{
tabLength = currentTabLength;
}
}
// create the new size
Size newTabSize = new Size(tabLength, tabControl1.ItemSize.Height);
tabControl1.ItemSize = newTabSize;
}
}
Screen shot of sample code from above:
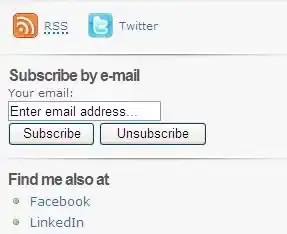