You can achieve this by using two forms. One with a partial opacity on the background and other with Transparency key on the foreground.
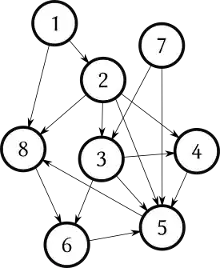
The transparent Window is in a Label kept on a form named Foreground_Form.
And the background is a form named Form_TransparentBack
Form_TransparentBack
public partial class Form_TransparentBack : Form
{
public Form_TransparentBack(Form _foregroundForm)
{
InitializeComponent();
StartPosition = _foregroundForm.StartPosition;
Location = _foregroundForm.Location;
Size = _foregroundForm.Size;
_foregroundForm.Resize += _foregroundForm_Resize;
FormBorderStyle = System.Windows.Forms.FormBorderStyle.None;
_foregroundForm.LocationChanged += _foregroundForm_LocationChanged;
ShowInTaskbar = false;
BackColor = Color.WhiteSmoke;
Opacity = 0.5;
Timer timer = new Timer() { Interval = 10};
timer.Tick += delegate(object sn, EventArgs ea)
{
(sn as Timer).Stop();
_foregroundForm.ShowDialog();
};
timer.Start();
Show();
}
void _foregroundForm_LocationChanged(object sender, EventArgs e)
{
Location = (sender as Form).Location;
}
void _foregroundForm_Resize(object sender, EventArgs e)
{
WindowState = (sender as Form).WindowState;
Size = (sender as Form).Size;
}
}
Foreground_Form
public partial class Foreground_Form : Form
{
public Foreground_Form()
{
InitializeComponent();
FormBorderStyle = System.Windows.Forms.FormBorderStyle.None; // required
TransparencyKey = this.BackColor; // required
StartPosition = FormStartPosition.CenterScreen;
this.Paint += Foreground_Form_Paint;
}
void Foreground_Form_Paint(object sender, PaintEventArgs e)
{
//this is for the Stroke
e.Graphics.DrawRectangle(Pens.White, new Rectangle(0, 0, Width - 1, Height - 1));
}
}
Now you can call any form with the transparent background. For the transparency, you should set the TransparencyKey to the form to be displayed.
private void button1_Click(object sender, EventArgs e)
{
new Form_TransparentBack(new Foreground_Form());
}