Networkx has a number of functions to draw graphs but also allow the user fine control over the whole process.
draw
is basic and its docstring specifically mentions:
Draw the graph as a simple representation with no nodeabels or edge
labels and using the full Matplotlib figure areas labels by default.
See draw_networkx() for more fatured drawing that allows title, axis
labels
The functions prefixed by draw_networkx
followed by edges
, nodes
, edge_labels
and edge_nodes
allow finer control over the whole drawing process.
Your example worked fine when using draw_networkx
.
In addition, if you are looking for an output that resembles an organogram, I would suggest the use of graphviz through networkx. Graphviz's dot
is ideal for this kind of diagrams (please also see this for dot).
In what follows, I have tried to modify your code slightly to demonstrate the use of both functions:
import networkx as nx
import matplotlib.pyplot as plt
import pandas
#Build the dataset
df = pandas.DataFrame({'emp_name':pandas.Series(['Marianne Becker', 'Evan Abbott', 'Jay Page', 'Seth Reese', 'Maxine Collier'], index=[0,1,2,3,4]), 'mgr_name':pandas.Series(['None', 'Marianne Becker', 'Marianne Becker', 'Marianne Becker', 'Marianne Becker'], index = [0,1,2,3,4])})
#Build the graph
G=nx.DiGraph()
G.add_nodes_from(df.emp_name)
G.nodes()
G.add_node('None')
#
#Over here, you are manually adding 'None' but in reality
#your nodes are the unique entries of the concatenated
#columns, i.e. emp_name, mgr_name. You could achieve this by
#doing something like
#
#G.add_nodes_from(list(set(list(D.emp_name.values) + list(D.mgr_name.values))))
#
# Which does exactly that, retrieves the contents of the two columns
#concatenates them and then selects the unique names by turning the
#combined list into a set.
#Add edges
subset = df[['mgr_name','emp_name']]
tuples = [tuple(x) for x in subset.values]
G.add_edges_from(tuples)
G.number_of_edges()
#Perform Graph Drawing
#A star network (sort of)
nx.draw_networkx(G)
plt.show()
t = raw_input()
#A tree network (sort of)
nx.draw_graphviz(G, prog = 'dot')
plt.show()
You could also try using graphviz's dot from the command line directly, by saving your networkx network via nx.write_dot
. To do this:
From within your python script:
nx.write_dot(G, 'test.dot')
After this, from your (linux) command line and assuming that you have graphviz installed:
dot test.dot -Tpng>test_output.png
feh test_output.png #Feh is just an image viewer.
firefox test_output.png & #In case you don't have feh installed.
For a more typical organogram format, you can force orthogonal edge routing by
dot test.dot -Tpng -Gsplines=ortho>test_output.png
Finally, here are the outputs
Output of draw_networkx
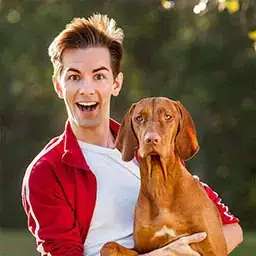
Output of draw_graphviz
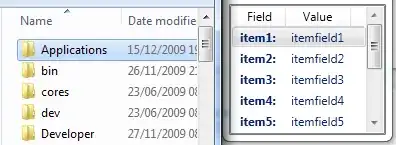
Output of dot
without orthogonal edges
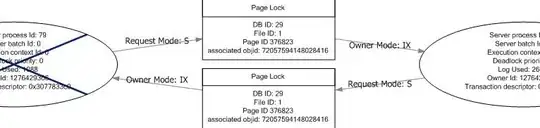
Output of dot
with orthogonal edges
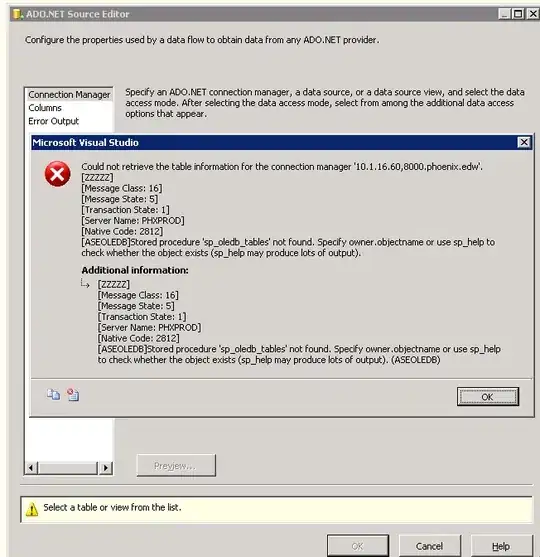
Hope this helps.