I assume by "tab" you mean the area highlighted in red:
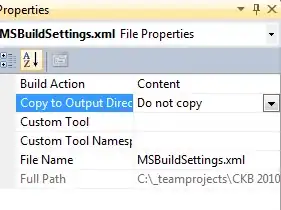
In all modern browsers a website cannot access anything out of its window
, except for APIs explicitly provided to it.
Therefore, there is not way for you to even access the tab bar with just JavaScript.
Whether or not there is at all a way to get access to the tab bar depends on the browser, but it will (most certainly) require a browser addon.
In Chrome, for example, this was not at all possible back in 2010 and it looks like nothing has changed there.
In Firefox however, an addon can actually do this.
Assuming you know how to attach a script to browser.xul, I'm leaving out install.rdf
, chrome.manifest
and overlay.xul
, so here's only the relevant JavaScript:
(function()
{
// Wait for the browser to settle
top.addEventListener('load', function load(event)
{
// It only needs to do that once
top.removeEventListener('load', load);
// Get notified about every page that loads
top.gBrowser.addEventListener('DOMContentLoaded', function(event)
{
// Get the current tab
var tab = top.gBrowser.mCurrentTab;
// Check if we already annoyified it
if(tab.annoyingOrange === undefined)
{
// If not, let's do that!
tab.annoyingOrange = 'Plumpkin';
// Add a mouseover event to it
top.gBrowser.mCurrentTab.addEventListener('mouseover', function(ev)
{
// Since we do that to all tabs, we need to check here if we're still the selected tab
if(ev.target == top.gBrowser.mCurrentTab)
{
// And now we can get onto everybody's nerves!
alert('Hey apple!!!');
}
});
}
});
});
})();
Tested with Firefox 37.0.1 on Windows.
[ Download .xpi ] (Protip: Unzip for source)
But if your browser does not support it, you are out of luck and there is nothing you can do!
Anyway, this is a very bad thing to do and it annoys people to no end!
This should never, never ever be done in a production or even beta environment!