You haven't actually said what the problem is in your question (is the form not submitting?) so this is going to be a very generalized answer on how to debug javascript problems such as this.
First, you should insert calls to console.log
to see what's going on better:
$(function() {
console.log('DOCUMENT READY'); // <<<<<<<<< ADD LOG HERE
$('.button-primary').click(function(event) {
console.log('CLICK EVENT WORKED'); // <<<<<<<<< ADD LOG HERE
var email = $('#emailInput').val();
var password = $('#passwordInput').val();
var firstName = $('#fnameInput').val();
var lastName = $('#lnameInput').val();
console.log('SENDING', email, password, firstname, lastname); // <<<<<<<<< ADD LOG HERE
$.post( 'http://<MY-DYNO>.herokuapp.com/api/v1/users/', { fname: firstName,
lname: lastName,
password: password,
email: email,
success: function(response) {
$('#registerStatus').text('Successfully registered');
console.log(response);
}
}).done(function() { console.log('your ajax call worked'); }) // <<<<<<<<< ADD LOG HERE
.fail(function() { console.log('something went wrong'); }) // <<<<<<<<< ADD LOG HERE
.always(function() { console.log('something went really wrong'); }); // <<<<<<<<< ADD LOG HERE
});
});
Now run this and see which logs get written (in Chrome go to right click webpage > inspect element > console
) - you'll now know how far your code gets before it stops working.
Another useful tool for debugging ajax requests (assuming again you're using Chrome) is the Network
tab within the debugging console. On your webpage right click > inspect element
and choose the Network
tab at the top. You'll see something like this:
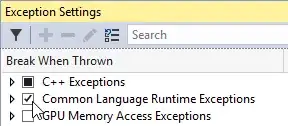
Make sure the circle in the top left is red and if not, press it. Now Chrome is monitoring web requests (including ajax requests) for your current webpage, and you can now submit your form and make sure that a new record is added to the top of this list of requests. You can then click on the request and see exactly to which URL it submitted to, what data was submitted, and what the response was.
You should also be using the Console
tab at the top as well. If your request was blocked because you're on domainA.com and did an AJAX request to domainB.com (this is illegal - you can't do AJAX cross domain without a CORS technique such as JSONP or without explicit CORS headers on the server) then such an error will show up in the console tab:
(note: the requests in the above image were blocked by adblock - but you get the picture, this tab will tell you if/why AJAX requests failed)
You should now know exactly what was preventing your AJAX request from working properly. If none of the console.log
calls ran then the problem is with your inclusion of jQuery, or your event listener on your button. If the 'SENDING' console.log
right before the AJAX call doesn't work then you know the problem has to do with extracting data from DOM elements on your webpage (or whatever is between the previous call to console.log and this one). If all these console.log
calls work then you should inspect the Network
tab and continue to look in the Console
tab in Chrome for more clues as to what went wrong, as at this point the problem is likely to do with you violating the same origin policy and thus having to do a technique like this to get around it, or the problem could just be with your server and you may want to consider debugging options there.
edit: in accordance with your edit where you've said the server isn't receiving what you're sending it, to confirm that this is actually true you should first check the Console
tab to see if the values you're sending out were printed (recall that we added a console.log call to print out firstName, lastName, etc). If you don't see those being printed out on your Console
tab then the problem has to do with your lines such as var firstName = $('#fnameInput').val();
being incorrect.
If you do see the right values for email, password, firstName and lastName being printed on the Console
tab, you then need to perform the actions outlined above where you record the request from the Network tab in Chrome (when you click on the request in the Network tab, in the 'Request Headers' or 'Form Data' section you should confirm that the variables you are sending are either there or not there). If you do see the variables being sent as part of the request then you should also make sure that you're submitting the right kind of request (POST
and not GET
). You should also confirm with your server code that you are sending the right names for variables ("email" not "Email"). If all this matches up then it's possible that the problem is with the server application.