Unfortunately, there is no such utility for IOS. I needed a similar solution, so I've come with the below.
The below function draws a left arrow and returns an UIImage. Note that one can draw the arrow by using only paths. Also, one can also use a pre created image from assets instead of creating all the time or create one re-use it.
func drawArrow() ->UIImage {
//the color of the arrow
let color = UIColor.yellow
// First create empty image choose the size according to your need.
let width = 84
let height = 24
let size = CGSize( width: width, height: height)
//let image: UIImage = makeEmptyImage(size: size)
let rectangle = CGRect(x: 12, y: 0, width: 72, height: 24)
UIGraphicsBeginImageContextWithOptions( size, false, 0.0)
let context = UIGraphicsGetCurrentContext()
//clear the background
context?.clear(CGRect(x: 0, y: 0, width: width, height: height))
//draw the rectangle
context?.addRect(rectangle)
context?.setFillColor(color.cgColor)
context?.fill(rectangle)
//draw the triangle
context?.beginPath()
context?.move( to: CGPoint(x : 12 ,y : 0) )
context?.addLine(to: CGPoint(x : 0, y : 12) )
context?.addLine(to: CGPoint(x : 12, y : 24) )
context?.closePath()
context?.setFillColor(color.cgColor)
context?.fillPath()
// get the image
let image2 = UIGraphicsGetImageFromCurrentImageContext()!
UIGraphicsEndImageContext()
return image2
}
The next function, takes the image and draw a text onto it.
func drawText(text:NSString, inImage:UIImage) -> UIImage? {
let font = UIFont.systemFont(ofSize: 11)
let color = UIColor.black
let style : NSMutableParagraphStyle = NSMutableParagraphStyle.default.mutableCopy() as! NSMutableParagraphStyle
style.alignment = .center
let attributes:NSDictionary = [ NSAttributedString.Key.font : font,
NSAttributedString.Key.paragraphStyle : style,
NSAttributedString.Key.foregroundColor : color
]
let myInsets = UIEdgeInsets(top: 0, left: 20, bottom: 0, right: 0)
let newImage = inImage.resizableImage(withCapInsets: myInsets)
print("rect.width \(inImage.size.width) rect.height \(inImage.size.height)")
let textSize = text.size(withAttributes: attributes as? [NSAttributedString.Key : Any] )
let textRect = CGRect(x: 10, y: 5, width: textSize.width, height: textSize.height)
UIGraphicsBeginImageContextWithOptions(CGSize(width: textSize.width + 20, height: textSize.height + 10), false, 0.0)
newImage.draw(in: CGRect(x: 0, y: 0, width: textSize.width + 20 , height: textSize.height + 10))
text.draw(in: textRect.integral, withAttributes: attributes as? [NSAttributedString.Key : Any] )
let resultImage = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
return resultImage
}
so we can use it like
marker.icon = drawText(text: eLoc.name as NSString, inImage: drawArrow() )
marker.groundAnchor = CGPoint(x: 0, y: 0.5)
//If you need some rotation
//let degrees = CLLocationDegrees(exactly: 90.0)
//marker.rotation = degrees!
groundAnchor
moves the position of the marker icon, see this anwser. With degree you can rotate the arrow.
Note : Unfortunately, IOS doesn't support 9-patch images. Therefore, not all of the bubble icon factory can be programmed by using resizableImage, see this nice web site and also see this answer
An example output is;
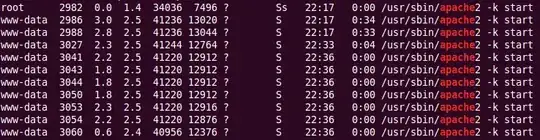