I had a situation to show the distance between two markers above the polyline.
Below is what was my requirement:
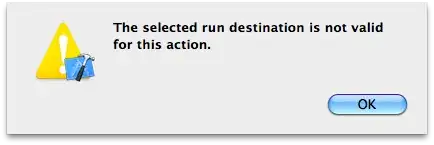
I managed to do it in some other way and it is quite interesting way, this way you can customize it to achieve anything.
Steps:
- Calculate the distance between the markers by using Location.distanceBetween() method.
- Create a new
Layout XML
file and create whatever type of UI
you want to show for your text. In my case it contained only one TextView
.
- Set the calculated distance in the
TextView
.
- Convert the
XML Layout
to Bitmap
.
- Create a
BitmapDescriptor
from that Bitmap
object.
- Find out the midpoint between the desired markers and add a custom marker by using the
BitmapDescriptor
created in last step and you are done.
Code:
To calculate distance between two markers
float[] distance1 = new float[1];
Location.distanceBetween(userMarker.getPosition().latitude, userMarker.getPosition().longitude, positionMarker.getPosition().latitude, positionMarker.getPosition().longitude, distance1);
Create Bitmap from XML Layout file
LinearLayout distanceMarkerLayout = (LinearLayout) getLayoutInflater().inflate(R.layout.distance_marker_layout, null);
distanceMarkerLayout.setDrawingCacheEnabled(true);
distanceMarkerLayout.measure(MeasureSpec.makeMeasureSpec(0, MeasureSpec.UNSPECIFIED), MeasureSpec.makeMeasureSpec(0, MeasureSpec.UNSPECIFIED));
distanceMarkerLayout.layout(0, 0, distanceMarkerLayout.getMeasuredWidth(), distanceMarkerLayout.getMeasuredHeight());
distanceMarkerLayout.buildDrawingCache(true);
TextView positionDistance = (TextView) distanceMarkerLayout.findViewById(R.id.positionDistance);
positionDistance.setText(distance1[0]+" meters");
Bitmap flagBitmap = Bitmap.createBitmap(distanceMarkerLayout.getDrawingCache());
distanceMarkerLayout.setDrawingCacheEnabled(false);
BitmapDescriptor flagBitmapDescriptor = BitmapDescriptorFactory.fromBitmap(flagBitmap);
To find out mid point between two markers do something like the following:
double dLon = Math.toRadians(flagMarker.getPosition().longitude - positionMarker.getPosition().longitude);
double lat1 = Math.toRadians(positionMarker.getPosition().latitude);
double lat2 = Math.toRadians(flagMarker.getPosition().latitude);
double lon1 = Math.toRadians(positionMarker.getPosition().longitude);
double Bx = Math.cos(lat2) * Math.cos(dLon);
double By = Math.cos(lat2) * Math.sin(dLon);
double lat3 = Math.atan2(Math.sin(lat1) + Math.sin(lat2), Math.sqrt((Math.cos(lat1) + Bx) * (Math.cos(lat1) + Bx) + By * By));
double lon3 = lon1 + Math.atan2(By, Math.cos(lat1) + Bx);
lat3 = Math.toDegrees(lat3);
lon3 = Math.toDegrees(lon3);
Add a new cutom marker by using the bitmap descriptor created by us in previous steps
Marker centerOneMarker = mMap.addMarker(new MarkerOptions()
.position(new LatLng(lat3, lon3))
.icon(flagBitmapDescriptor));
Hope it helps you.