You can use XElement.Parse
to get the node value like this:
var htmlString = "<p>First Sentence is this. Second sentence is this.</p>";
var result = System.Xml.Linq.XElement.Parse(htmlString).Value;
If not all the strings contain valid XML structure, or may have no tags at all, you can add fake tags like this:
var htmlString = "<p>First Sentence is this. Second sentence is this.</p>";
var result = System.Xml.Linq.XElement.Parse("<root>" + htmlString + "</root>").Value;
Result:
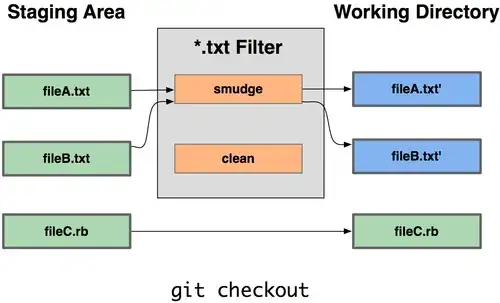
You might want to add error handling for this, but this is clearly better than using a regex for this.
EDIT:
In case this is still not working, and you want to just handle the entities, you can leverage System.Web.HttpUtility.HtmlDecode
method to replace HTML entities with literals:
var final_result = System.Web.HttpUtility.HtmlDecode(result);