Your question says,
Loop through all items in a listview.
I understand from your code that you want to add the items from String array
to ArrayList
.
But, you can pass String array directly as a third parameter to your ArrayAdapter
.
Look at the suggestions provided by Android studio for ArrayAdapter
. You can pass String[]
or ArrayList
too :
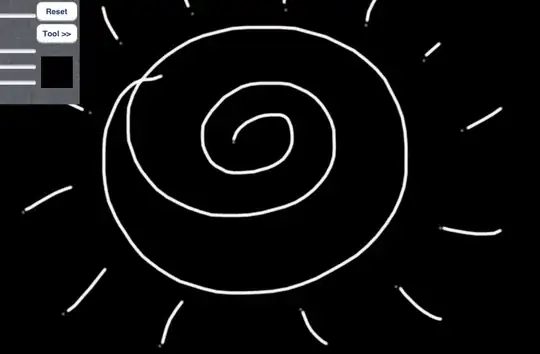
Either you can pass String[] or if you wanted to loop through all String[] items to ArrayList, you can simply do by a single line.
Collections.addAll(arrayList,values);
arrayList - ArrayList
values - String[]
instead of,
listItems = new ArrayList<String>();
for (int i = 0; i < values.length; ++i) {
listItems.add(values[i]);
}
And in comment section, you said
I think I may add/remove item at a time to the listView
later.
In this case, you can have some button to reload the list to show the old items + added new items or to show the list except the items which you've deleted. I'll add below how you have to achieve it.
Have a button AddMore
in your layout and whenever you want to add new items, then do like this
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
arrayList.add("lemon"); // this adds item lemon to arraylist
arrayList.add("Pomgranete");
arrayAdapter.notifyDataSetChanged(); // this will refresh the listview and shows the newly added items too
}
});
You can delete the item similarly by passing the position of the item in arrayList,
arrayList.remove(arrayList.get(i)); // i is the position & note arrayList starts from 0
So, by summing up everything, here's the full working code :
public class MainActivity extends AppCompatActivity {
ListView listView;
String[] values = {"Apple", "Orange", "Banana"};
List<String> arrayList;
Button button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
listView = (ListView)findViewById(R.id.listView);
button = (Button)findViewById(R.id.button);
arrayList = new ArrayList<String>();
Collections.addAll(arrayList,values); // here you're copying all items from String[] to ArrayList
final ArrayAdapter<String> arrayAdapter = new ArrayAdapter<String>(this,android.R.layout.simple_list_item_1,arrayList);
listView.setAdapter(arrayAdapter);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
arrayList.remove(arrayList.get(2)); // here i remove banana since it's position is two. My ordering of items is different so it removed banana. If i use ordering from your quest, it will remove orange.
arrayList.add("lemon"); // adding lemon to list
arrayList.add("Pomgranete"); // adding pomgranete
arrayAdapter.notifyDataSetChanged(); // this used to refresh the listView
}
});
}
}
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent" tools:context=".MainActivity">
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="ADD MORE"
android:layout_alignParentBottom="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:id="@+id/button" />
<ListView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/listView"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:layout_above="@+id/button" />
</RelativeLayout>
Output :
List with pre-defined 3 items and one button to load more items.

List with old 3 items + newly added 2 items (Here i didn't use arrayList.remove)
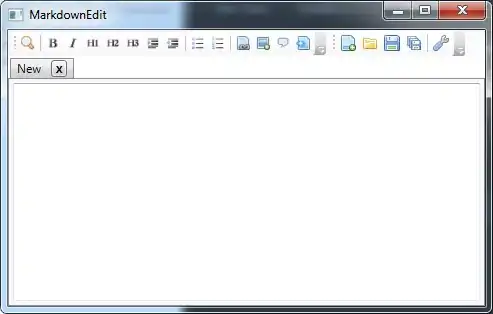
List with old items except deleted item + newly added 2 items (Here i used arrayList.remove to remove banana by arrayList.remove(arrayList.get(2));
)
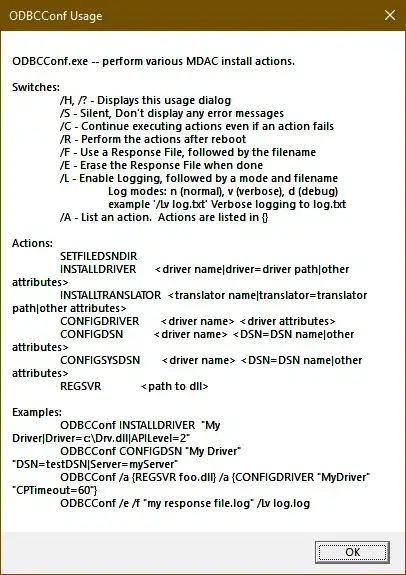