You can stack an ImageView
on top of a Canvas
. Use a StackPane
. You can later add mouse listeners to the ImageView
.
Is there any possibilities with or without ImageView
(buttons or any controls?) or is it restricted to use controls over canvas?
All controls including Button
, ImageView
and Canvas
itself extend from Node
and can be used to add in the StackPane
.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.image.ImageView;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class Main extends Application {
int count = 1;
@Override
public void start(Stage stage) {
StackPane root = new StackPane();
Scene s = new Scene(root, 400, 400, Color.BLACK);
final Canvas canvas = new Canvas(300, 300);
GraphicsContext gc = canvas.getGraphicsContext2D();
gc.setFill(Color.BLUE);
gc.fillRect(10, 10, 300, 300);
ImageView image = new ImageView("https://cdn0.iconfinder.com/data/icons/toys/256/teddy_bear_toy_6.png");
// Listener for MouseClick
image.setOnMouseClicked(e -> {
Stage popup = new Stage();
popup.initOwner(stage);
popup.show();
});
root.getChildren().addAll(canvas, image);
stage.setScene(s);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Output:
- Scene has Black Background
- Canvas has Blue Background
- ImageView is the bear.
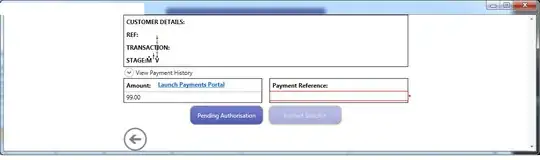