If you need any more information post it in the comment below.
First you want to add the library to the project in the gradle or install the library.
Gradle add compile 'com.jpardogo.materialtabstrip:library:1.0.9' or download project at https://github.com/jpardogo/PagerSlidingTabStrip
apply plugin: 'com.android.application'
android {
compileSdkVersion 22
buildToolsVersion "22.0.1"
defaultConfig {
applicationId "com.eugene.pagertesting"
minSdkVersion 14
targetSdkVersion 22
versionCode 1
versionName "1.0"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
}
}
}
dependencies {
compile fileTree(dir: 'libs', include: ['*.jar'])
compile 'com.android.support:appcompat-v7:22.1.1'
compile 'com.jpardogo.materialtabstrip:library:1.0.9'
}
XML
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/primary"
android:minHeight="56dp"
app:theme="@style/ThemeOverlay.AppCompat.Dark"/>
<com.astuetz.PagerSlidingTabStrip
android:id="@+id/tabs"
android:layout_width="match_parent"
android:layout_height="48dp"
android:layout_below="@+id/toolbar"
android:background="@color/primary"
android:textColorPrimary="@color/white"
app:pstsDividerColor="@color/primary"
app:pstsIndicatorColor="@color/white"
app:pstsIndicatorHeight="2dp"
app:pstsShouldExpand="true"
app:pstsUnderlineHeight="0dp"/>
<android.support.v4.view.ViewPager
android:id="@+id/pager"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_below="@+id/tabs"/>
</RelativeLayout>
PagerAdapter
public class PagerAdapter extends FragmentPagerAdapter {
public PagerAdapter(FragmentManager fm) {
super(fm);
}
private Fragment f = null;
@Override
public Fragment getItem(int position) { // Returns Fragment based on position
switch (position) {
case 0:
f = new FragmentPageOne();
break;
case 1:
f = new FragmentPageTwo();
break;
}
return f;
}
@Override
public int getCount() { // Return the number of pages
return 2;
}
@Override
public CharSequence getPageTitle(int position) { // Set the tab text
if (position == 0) {
return "Fragment One";
}
if (position == 1) {
return "Fragment Two";
}
return getPageTitle(position);
}
}
MainActivity
public class MainActivity extends AppCompatActivity {
ViewPager viewPager;
PagerAdapter pagerAdapter;
PagerSlidingTabStrip pagerSlidingTabStrip;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
viewPager = (ViewPager) findViewById(R.id.pager);
pagerAdapter = new PagerAdapter(getSupportFragmentManager());
pagerSlidingTabStrip = (PagerSlidingTabStrip) findViewById(R.id.tabs);
viewPager.setAdapter(pagerAdapter);
pagerSlidingTabStrip.setViewPager(viewPager);
}
}
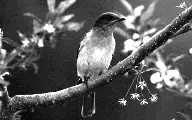
If you want to know how I styled the app I will post that as well just let me know.
UPDATE
if you would like to drop the shadow below the tabs I can post that as well.