You can create a custom PrintStream
that inspects the current stack trace and includes file / line numbers in the output. You can then use System.setOut
/System.setErr
to cause all stdout/stderr output to include this information.
By formatting it properly Eclipse will pick it up as stack trace elements and generate links.
Here's a complete demo:
public class Main {
public static void main(String args[]) {
System.setOut(new java.io.PrintStream(System.out) {
private StackTraceElement getCallSite() {
for (StackTraceElement e : Thread.currentThread()
.getStackTrace())
if (!e.getMethodName().equals("getStackTrace")
&& !e.getClassName().equals(getClass().getName()))
return e;
return null;
}
@Override
public void println(String s) {
println((Object) s);
}
@Override
public void println(Object o) {
StackTraceElement e = getCallSite();
String callSite = e == null ? "??" :
String.format("%s.%s(%s:%d)",
e.getClassName(),
e.getMethodName(),
e.getFileName(),
e.getLineNumber());
super.println(o + "\t\tat " + callSite);
}
});
System.out.println("Hello world");
printStuff();
}
public static void printStuff() {
System.out.println("More output!");
}
}
Eclipse Console:
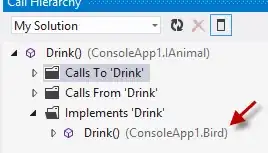
I consider this to be a hack though, and I wouldn't use it for anything but casual debugging.