Using bsxfun
, permute
and calculating Euclidean distance based on native approach
This may not look elegant, but its very faster than pdist2
for small data sizes
d = sqrt(sum((bsxfun(@minus,permute(A,[1 3 2]),permute(B,[3 1 2]))).^2,3));
[~, iB] = min(d); %// from Luis's answer
[~, iA] = min(d.');
Bechmarking (performed after warming up several times)
tic
out = sqrt(sum((bsxfun(@minus,permute(A,[1 3 2]),permute(B,[3 1 2]))).^2,3));
toc
tic
d = pdist2(A,B);
toc
Elapsed time is 0.000080 seconds.
Elapsed time is 0.000453 seconds.
Bench Marking performed (using timeit()
)for Different data sizes of A
keeping Datasize of B
constant at 20.
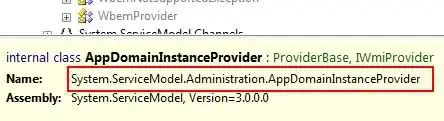
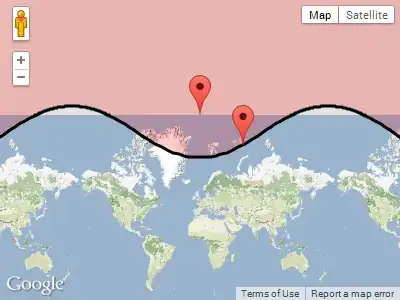
Conclusion:
pdist2
is significantly efficient with large datasizes while bsxfun
+permute
could be used for very small data sizes.