You can achieve this easily by using react-native-easy-grid library.
As it is a 2X2 layout, you can use it the following way. First import the library
import {Grid,Row,Col} from 'react-native-easy-grid'
Code:
export default class StackOverflow extends Component {
constructor(props) {
super(props);
const ds = new ListView.DataSource({
rowHasChanged: (r1, r2) => r1 !== r2
});
this.state = {
dataSource: ds.cloneWithRows(["item"])
};
}
render() {
return (
<View style={{ flex: 1, padding: 50 }}>
<ListView
dataSource={this.state.dataSource}
renderRow={rowData => (
<Grid>
<Row style={styles.container}>
<Col
style={[
styles.container,
{ backgroundColor: "yellow" }
]}
>
<Text>{rowData}</Text>
</Col>
<Col
style={[
styles.container,
{ backgroundColor: "green" }
]}
>
<Text>{rowData}</Text>
</Col>
</Row>
<Row style={styles.container}>
<Col
style={[
styles.container,
{ backgroundColor: "skyblue" }
]}
>
<Text>{rowData}</Text>
</Col>
<Col
style={[
styles.container,
{ backgroundColor: "orange" }
]}
>
<Text>{rowData}</Text>
</Col>
</Row>
</Grid>
)}
/>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
alignItems: "center",
justifyContent: "center"
}
});
The result would be this :
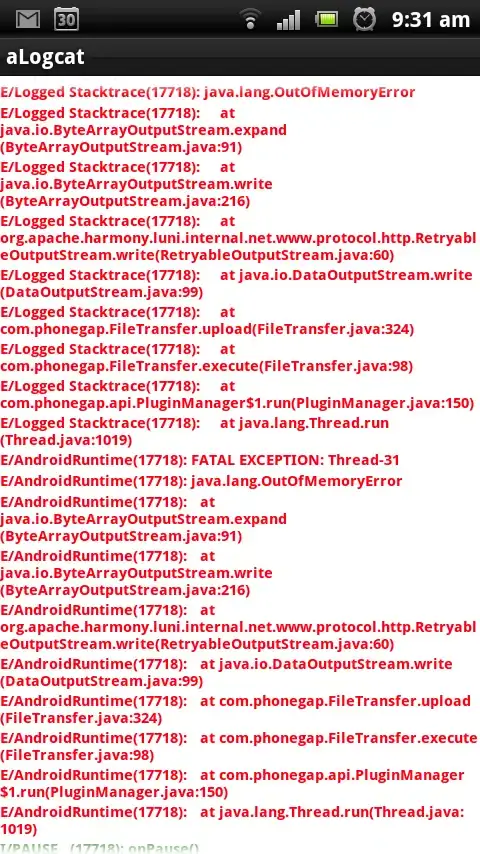
Explaination:
I created a 2X2 layout using the react-native-easy-grid library using the components Grid, Row, Col. The beauty is you need not even mention any flexDirection attributes, it just works. Then, I passed this component to the renderRow prop of ListView element.