An easy way to achieve this is to use a single Document
for both text fields. The Document
is the model that stores the data (in this case, plain text).
Here is a simple example showing two text fields, one in a frame and the other in an option pane. Update the text in the field in the dialog to see the other text field update instantly.
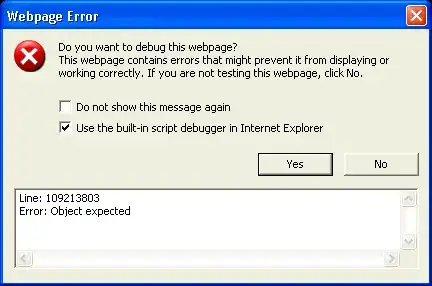
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import javax.swing.border.EmptyBorder;
import javax.swing.text.Document;
public class TwoFieldsUsingOneDocument {
private JComponent ui = null;
TwoFieldsUsingOneDocument() {
initUI();
}
public void initUI() {
if (ui!=null) return;
ui = new JPanel(new BorderLayout(4,4));
ui.setBorder(new EmptyBorder(4,4,4,4));
JTextField frameTextField = new JTextField(15);
Document doc = frameTextField.getDocument();
final JTextField dialogTextField = new JTextField(15);
// get both fields to use a single Document
dialogTextField.setDocument(doc);
final JButton showInputDialog = new JButton("Get Input!");
showInputDialog.setMargin(new Insets(50, 120, 50, 120));
ActionListener showInputActionListener = new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JOptionPane.showMessageDialog(
showInputDialog,
dialogTextField,
"Change text",
JOptionPane.QUESTION_MESSAGE);
}
};
showInputDialog.addActionListener(showInputActionListener);
ui.add(showInputDialog);
ui.add(frameTextField, BorderLayout.PAGE_END);
}
public JComponent getUI() {
return ui;
}
public static void main(String[] args) {
Runnable r = new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (Exception useDefault) {
}
TwoFieldsUsingOneDocument o = new TwoFieldsUsingOneDocument();
JFrame f = new JFrame(o.getClass().getSimpleName());
f.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
f.setLocationByPlatform(true);
f.setContentPane(o.getUI());
f.pack();
f.setMinimumSize(f.getSize());
f.setVisible(true);
}
};
SwingUtilities.invokeLater(r);
}
}