Improving on Stehpen's answer above...
Assume you've added a documents folder to your project:
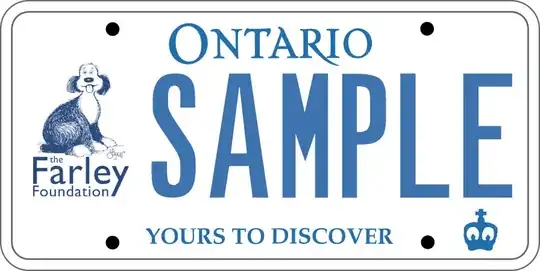
Create a method GetDocument where the GetFilePath method refers to the Folder/Filename in the folder above.
private void GetDocument()
{
string fileName = Environment.CurrentDirectory.GetFilePath("Documents\\Title.xps");
Debugger.Break();
XpsDocument doc = new XpsDocument(fileName, FileAccess.Read);
XDocViewer.Document = doc.GetFixedDocumentSequence();
}
Where GetFilePath is an extension method that looks like this:
public static class StringExtensions
{
public static string GetFilePath(
this string EnvironmentCurrentDirectory, string FolderAndFileName)
{
//Split on path characters
var CurrentDirectory =
EnvironmentCurrentDirectory
.Split("\\".ToArray())
.ToList();
//Get rid of bin/debug (last two folders)
var CurrentDirectoryNoBinDebugFolder =
CurrentDirectory
.Take(CurrentDirectory.Count() - 2)
.ToList();
//Convert list above to array for Join
var JoinableStringArray =
CurrentDirectoryNoBinDebugFolder.ToArray();
//Join and add folder filename passed in
var RejoinedString =
string.Join("\\", JoinableStringArray) + "\\";
var final = RejoinedString + FolderAndFileName;
return final;
}
}