The simple answer as to why is returns false
is because even though the functions do the same thing and look the same, they are initialized in two different memory locations (for objects like functions, plain objects, and arrays, the strict equality operator ===
checks the memory location of that object.)
Also, if you're going to use Node.js, you have to make sure it is interpreting it as ECMAScript6 (otherwise, () => 0
is not valid ES5, which is default for Node.js).
You can use the --harmony
flag:
node --harmony app.js
See this question about "What does node --harmony
do?" for more info about using ES6 in Node.js. A brief quote from the top answer:
it seems harmony enables new ECMA features in the language. The reason
your file won't run without harmony is because app.js is probably
using non-backward compatible features from the new ECMA standard
(like block scoping, proxies, sets, maps, etc.)
To explain why you might be seeing the three dots, see this question:
So basically, you opened node in an interactive terminal, and then
typed node example.js, so it is trying to run that as if it were
JavaScript. It shows the three dots because that is not valid
JavaScript code, and it is waiting for you to type more code that
might make it valid.
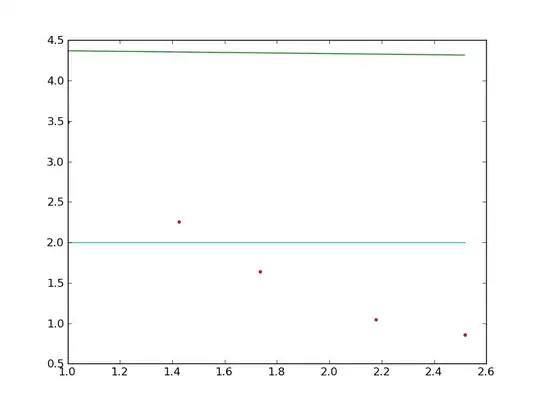
The above is my output. This is what I ran in my terminal (using 0.12.21
):
> $ node --harmony
> (() => 0) === (() => 0)
> false