From the documentation, the .fit()
method returns:
shape, loc, scale : tuple of floats
MLEs for any shape statistics, followed by those for location and scale.
and the .pdf()
method accepts:
x : array_like
quantiles
arg1, arg2, arg3,... : array_like
The shape parameter(s) for the distribution (see docstring of the instance object for more information)
loc : array_like, optional
location parameter (default=0)
scale : array_like, optional
So essentially you would do something like this:
import numpy as np
from scipy import stats
from matplotlib import pyplot as plt
# some random variates drawn from a beta distribution
rvs = stats.beta.rvs(2, 5, loc=0, scale=1, size=1000)
# estimate distribution parameters, in this case (a, b, loc, scale)
params = stats.beta.fit(rvs)
# evaluate PDF
x = np.linspace(0, 1, 1000)
pdf = stats.beta.pdf(x, *params)
# plot
fig, ax = plt.subplots(1, 1)
ax.hold(True)
ax.hist(rvs, normed=True)
ax.plot(x, pdf, '--r')
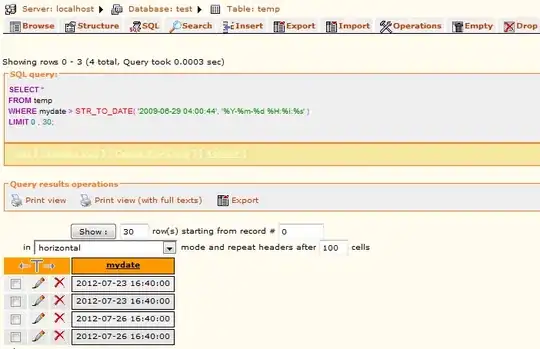