Your request of an "aesthetically pleasing" graph is a little vague, but here is how you could produce a labelled and colourful plot using ggplot2.
First simulate some data to fit the format you describe:
set.seed(2015)
df = data.frame(week = 1:5,
pct1 = sample(1:100, 5),
pct2 = sample(1:100, 5),
pct3 = sample(1:100, 5),
pct4 = sample(1:100, 5),
pct5 = sample(1:100, 5))
df
week pct1 pct2 pct3 pct4 pct5
1 1 7 36 71 89 70
2 2 84 50 39 27 41
3 3 30 8 4 8 21
4 4 4 64 40 79 65
5 5 14 99 72 37 71
To produce the desired plot with ggplot2, you should convert the data to a "long" format. I use the function gather
from the package tidyr
(you could also use the equivalent melt
function from package reshape2
).
library(tidyr)
library(ggplot2)
# Gather the data.frame to long format
df_gathered = gather(df, pct_type, pct_values, pct1:pct5)
head(df_gathered)
week pct_type pct_values
1 1 pct1 7
2 2 pct1 84
3 3 pct1 30
4 4 pct1 4
5 5 pct1 14
6 1 pct2 36
Now you can easily produce a plot by colouring using the pct_type
variable.
ggplot(data = df_gathered, aes(x = week, y = pct_values, colour = pct_type)) +
geom_point() + geom_line(size = 1) + xlab("Week index") + ylab("Whatever (%)")
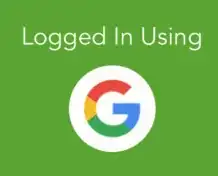
Note:
In case the variable week
is a factor (I assumed it was a number, as you referred to it as "week index"), you would also need to tell ggplot
to group data by pct_type
, like so:
ggplot(data = df_gathered, aes(x = week, y = pct_values,
colour = pct_type, group = pct_type)) +
geom_point() + geom_line(size = 1) + xlab("Week index") + ylab("Whatever (%)")