You can do it with ImageMagick which is installed on most Linux distros and is available for free for OSX (ideally via homebrew
) and also for Windows from here.
If you start with this smooth greyscale ramp:
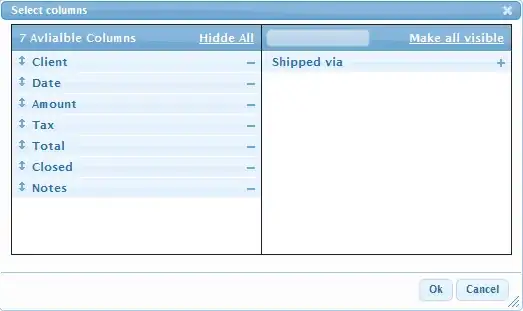
At the command line, you can use this for Floyd-Steinberg dithering:
convert grey.png -dither FloydSteinberg -monochrome fs.bmp
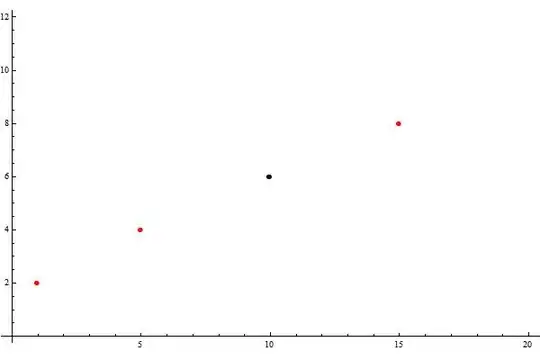
or, this for Riemersma dithering:
convert grey.png -dither Riemersma -monochrome riem.bmp
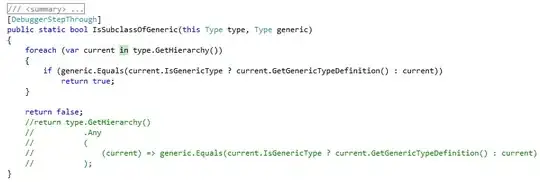
The Ordered Dither that Glenn was referring to is available like this with differing tile options:
convert grey.png -ordered-dither o8x8 -monochrome od8.bmp
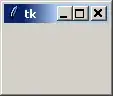
convert grey.png -ordered-dither o2x2 -monochrome od2.bmp
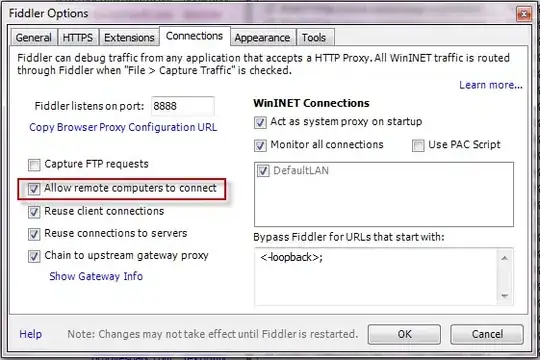
A check of the format shows it is 1bpp with a 2-colour palette:
identify -verbose riem.bmp
Image: riem.bmp
Format: BMP (Microsoft Windows bitmap image)
Class: PseudoClass
Geometry: 262x86+0+0
Units: PixelsPerCentimeter
Type: Bilevel
Base type: Bilevel <--- 1 bpp
Endianess: Undefined
Colorspace: Gray
Depth: 1-bit <--- 1 bpp
Channel depth:
gray: 1-bit
Channel statistics:
Pixels: 22532
Gray:
min: 0 (0)
max: 1 (1)
mean: 0.470486 (0.470486)
standard deviation: 0.499128 (0.499128)
kurtosis: -1.98601
skewness: 0.118261
entropy: 0.997485
Colors: 2
Histogram:
11931: ( 0, 0, 0) #000000 gray(0)
10601: (255,255,255) #FFFFFF gray(255)
Colormap entries: 2
Colormap:
0: ( 0, 0, 0) #000000 gray(0) <--- colourmap has only black...
1: (255,255,255) #FFFFFF gray(255) <--- ... and white
If you start with a colour image like this:
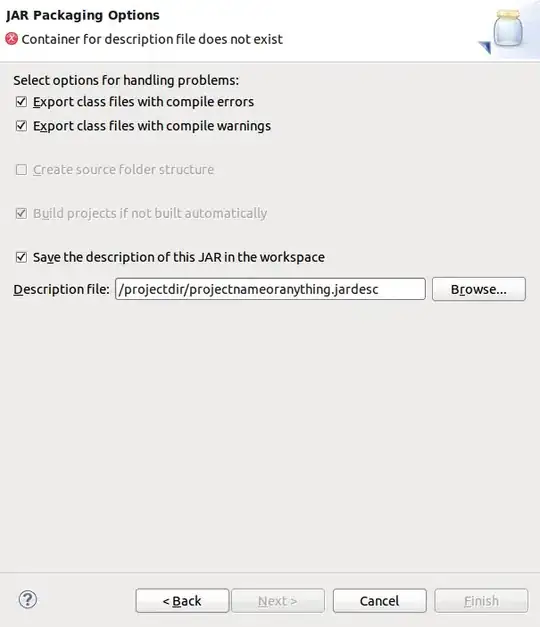
and process like this:
convert colour.png -ordered-dither o8x8 -monochrome od8.bmp
you will get this

As Glenn says, there are C# bindings for ImageMagick - or you can just use the above commands in a batch file, or use the C# equivalent of the system()
call to execute the above ImageMagick commands.