Instead of hardcoding the timezone with GMT-04:00 use the literal expression of your geographic area (aka Canonical ID) as defined in this standard : Joda.org
this is what Google Apps Script is using.
You can also get that more simply using
SpreadsheetApp.getActive().getSpreadsheetTimeZone()
or from the calendar object : calendar.getTimeZone()
all these parameters will handle daylight savings automatically, which is not the case for the GMT+xx:xx method.
You shouldn't have any problem as long as both are on the same timezone. Calendars have a setTimeZone(timeZone)
method and spreadsheets have a setSpreadsheetTimeZone(timeZone)
method so it is easy to synchronize both
EDIT
Following comment
Since you seem to combine date and time in a unique date object and that the daylight saving puts you into trouble (because "pure time" values in spreadsheets are converted to a date JavaScript object automatically and with summer time correction), below is a small code that I use to workaround this issue. I added a few logs to show intermediate values;
function combineDateAndTime(){
// in this example cell A1 has a date and cell B1 has time
var sh = SpreadsheetApp.getActiveSpreadsheet().getSheets()[0];
var dateOnly = sh.getRange('A1').getValue();
var timeOnly = sh.getRange('B1').getValue();
Logger.log('dateOnly object is : '+dateOnly+'\ntimeOnly object is : '+timeOnly);
var hours = Number(Utilities.formatDate(timeOnly, 'GMT'+timeOnly.toString().split('GMT')[1],'hh'));
var minutes = timeOnly.getMinutes();
var completeDateAndTime = new Date(dateOnly.setHours(hours,minutes,0,0));
Logger.log('completeDateAndTime is : '+completeDateAndTime);
}
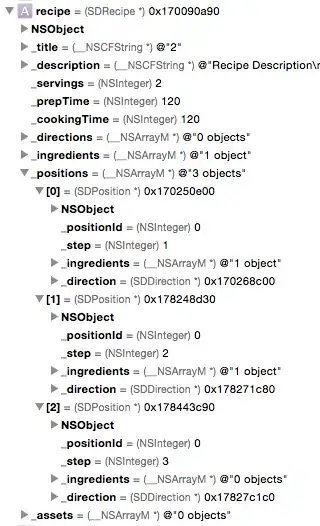
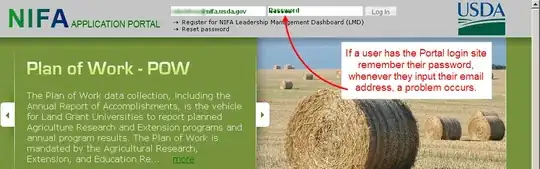
Note : there are other ways to retrieve hour values, see this post for example.
var time1 = new Date(values[1]);
Logger.log('time1: ' + time1);
// I will get:
// time1: Sat Dec 30 1899 09:30:00 GMT-0500 (EST)
When I add events using this time to the calendar, it becomes 10:30, because the calendar is GMT-0400 (EDT). – BrianL Jul 01 '15 at 15:02