You don't seem to actually use the time in your plot, but the issue is the longitudes wrapping around -180/180. This can be solved using coord_map
rather than coord_polar
and ensuring that the longitudes don't wrap around.
Load packages and generate sample data
library("ggplot2")
library("dplyr")
south_map <- map_data("world") %>% group_by(group) %>% filter(min(lat) <= -20)
set.seed(123)
track <- data.frame(long = cumsum(c(210,
unlist(lapply(c(1, -1), function(x) {
rnorm(50, x * 4, 4)
})))) %% 360 - 180,
lat = cumsum(c(-50, rnorm(100, 0.4, 2))),
A1 = sample(1:3, 101, replace = TRUE))
Ensure that coordinates don't wrap around:
track_new <- track
long_diff <- diff(track$long)
long_diff[long_diff < -180] <- long_diff[long_diff < -180] + 360
long_diff[long_diff > 180] <- long_diff[long_diff > 180] - 360
track_new$long <- cumsum(c(track$long[1], long_diff))
Plot using aziequidistant projection. Note that this assumes the North Pole in the centre, so the latitudes are flipped and then corrected with the scale.
ggplot(track_new, aes(x = long, y = -lat)) +
geom_polygon(aes(group = group), data = south_map, colour = "grey", fill = "gainsboro") +
coord_map("azequidistant") +
geom_point(aes(colour = factor(A1)), size = 2) +
geom_path(colour = "grey", size = 1) +
scale_x_continuous(breaks = NULL) +
scale_y_continuous("latitude", breaks = 25 * 0:3, labels = -25 * 0:3)
Final plot:
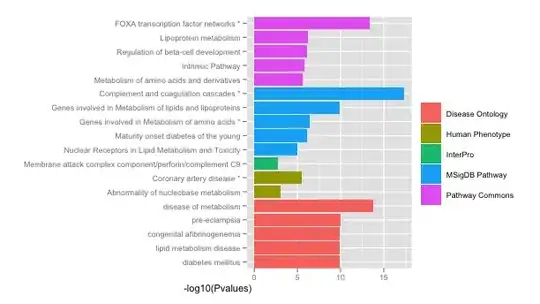
Just for interest, I thought it would be fun to produce an animation of this image. Here's the code to do it:
track_new$alpha <- 1
# Setup longitude labels
long_labels <- data.frame(long = 45 * -3:4, lat = -22.5)
long_labels$label <- long_labels$long
long_labels$label[8] <- "\U00B1 180"
long_labels$angle <- long_labels$long + 67.5 + 180 * (long_labels$long >= 45)
# Set up the basic plot
p <- ggplot(track_new, aes(x = long, y = -lat)) +
geom_polygon(aes(group = group), data = south_map, colour = "grey", fill = "gainsboro") +
coord_map("azequidistant", ylim = c(20, 90)) +
geom_point(aes(colour = A1, alpha = alpha), size = 2) +
geom_path(aes(alpha = alpha), colour = "grey", size = 1) +
scale_x_continuous(breaks = NULL) +
scale_y_continuous("latitude", breaks = 22.5 * 0:3, labels = -22.5 * 0:3) +
scale_alpha_identity(guide = "none") +
geom_text(aes(label = label, angle = angle),
data = long_labels, colour = "dark blue", alpha = 0.5, size = 4)
# Produce the animation
p$data$alpha <- 0
for(i in 1:(nrow(track_new) + 10)) {
p$data$alpha <- pmax(p$data$alpha - 0.1, 0)
if (i <= nrow(track_new)) {
p$data$alpha[i] <- 1
}
png(file.path("BirdPlots", sprintf("BirdPlot%03d.png", i)), width = 1024, height = 1024, res = 100)
print(p)
dev.off()
if (!(i %% 5)) cat(i, "\n")
}
# This needs ImageMagick in the system path. For non-Windows systems, you
# might be better using system rather than shell
shell(paste("convert", file.path("BirdPlots", "BirdPlot*.png"),
file.path("BirdPlots", "BirdPlotAnimation.gif")))
And here's the result:
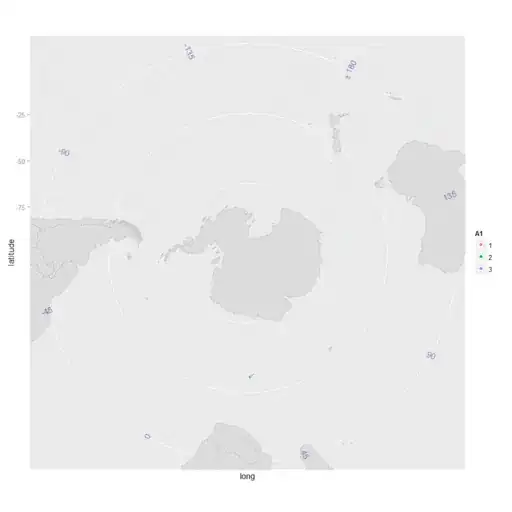
EDIT Corrected version of ayush's code
track_df2 <- new_df2
long_diff <- diff(new_df2$Longitude)
long_diff[long_diff < -180] <- long_diff[long_diff < -180] + 360
long_diff[long_diff > 180] <- long_diff[long_diff > 180] - 360
track_df2$Longitude <- cumsum(c(new_df2$Longitude[1], long_diff))
track_df2$a3_id <- factor(track_df2$a3_id)
ggplot(track_df2, aes(x = Longitude, y = -Latitude)) +
coord_map("azequidistant", ylim = c(20, 90)) +
geom_point(aes(colour = a3_id, alpha = alpha), size = 2) +
geom_path(aes(alpha = alpha), colour = "grey", size = 1) +
scale_x_continuous(breaks = NULL) +
scale_y_continuous(breaks = 22.5 * 0:3, labels = -22.5 * 0:3) +
scale_alpha_identity(guide = "none")