As you mentioned, prompt
works for browsers all the way back to IE:
var answer = prompt('question', 'defaultAnswer');
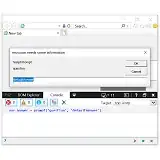
For Node.js > v7.6, you can use console-read-write
, which is a wrapper around the low-level readline
module:
const io = require('console-read-write');
async function main() {
// Simple readline scenario
io.write('I will echo whatever you write!');
io.write(await io.read());
// Simple question scenario
io.write(`hello ${await io.ask('Who are you?')}!`);
// Since you are not blocking the IO, you can go wild with while loops!
let saidHi = false;
while (!saidHi) {
io.write('Say hi or I will repeat...');
saidHi = await io.read() === 'hi';
}
io.write('Thanks! Now you may leave.');
}
main();
// I will echo whatever you write!
// > ok
// ok
// Who are you? someone
// hello someone!
// Say hi or I will repeat...
// > no
// Say hi or I will repeat...
// > ok
// Say hi or I will repeat...
// > hi
// Thanks! Now you may leave.
Disclosure I'm author and maintainer of console-read-write
For SpiderMonkey, simple readline
as suggested by @MooGoo and @Zaz.