Just for completeness since this has no answer and give an explanation.
To achieve compiling with C++ 11 (or another version) and Eclipse actually supporting it you need to do two things.
First the compiler flag needs to set so -std=c++11
or -std=c++0x
is appended when calling g++ or whatever is used.
- Open the properties for the properties for the project. Select it and right click ↦ Properties (or Alt+Enter for Windows users)
- Go to
C/C++ Build
↦ Settings
, maybe select your preferred configuration, ↦ GCC C++ Compiler
(or any other compiler you use) ↦ Dialect
.
- Select from the combo or write the flag to the
Other dialect
flags if not present in the combo (like -std=gnu++14
or -std=c++1z
).
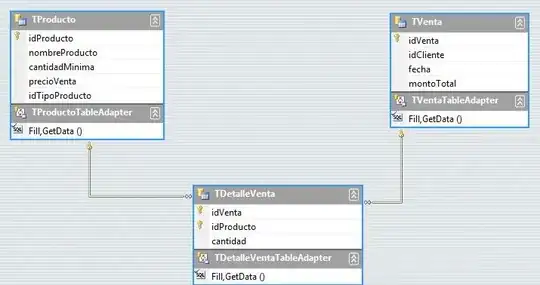
CDT will now call your compiler with -std=c++0x
when compiling. Now to the part so CDT supports C++11 and does not show errors for missing types and such. My libstdc++ contains lines like
#if __cplusplus < 201103L
# include <bits/c++0x_warning.h>
#else // C++0x
that causes your errors and the actual type declarations/definitions are greyed out if you view them in the C/C++ editor. __cplusplus
needs to be set correctly with #define __cplusplus 201103L
so they will be parsed and indexed by CDT. This is also done via the project settings or can also be done for the whole workspace.
- Go again to the project settings.
C/C++ General
↦ Preprocessor Include Paths, Macros etc.
, also maybe select your preferred configuration, ↦ tab Providers
.
- Select the entry for your compiler (for me it’s
CDT GCC Built-in Compiler Settings MinGW
.
- Add
-std=c++11
or -std=c++0x
to the Command to get compiler specs
textfield at any location.
- Optional: Select
Allocate console in the Console View
and hit apply. You should now see something like #define __cplusplus 201103L
in the console.
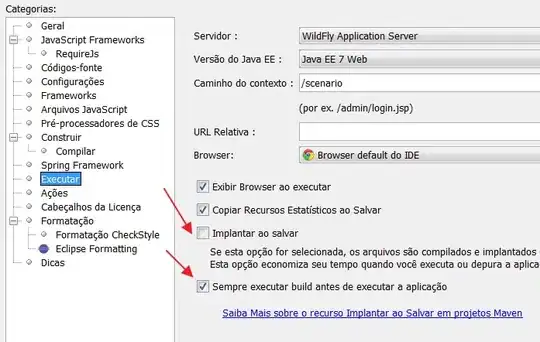
To set it for the whole workspace just check Use global provider shared between projects
and click on Workspace Settings
where an almost identical dialog opens.
I’m currently writing a plug-in that extends the new C/C++ project wizard where one can select the C++ version for the project that sets the compiler flag correctly and also the above setting for the indexer and some other stuff. But dunno if it will ever will be integrated into CDT or needs to be installed via plug-in. But it sure will be in https://www.cevelop.com in a few months.