First you need to understand how the Flexible box structure works. The below image is part of the Cheatsheet.
Flexbox Structure
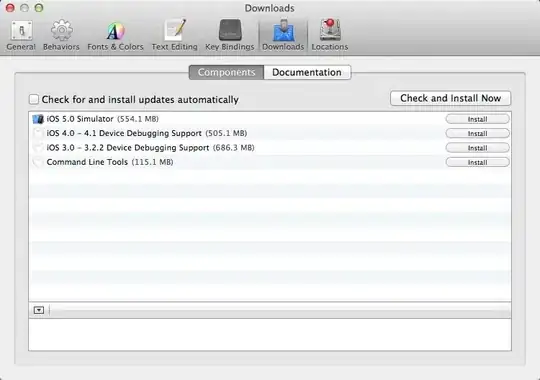
Flexbox was built to adapt both as a row and column.
Main-axis:
When flex-direction is row: the x-axis as on a graph. When flex-direction is column: the y-axis on a graph
Cross-axis:
When flex-direction is column: the x-axis as on a graph. When flex-direction is row: the y-axis on a graph
Justify-Content
justify-content
is used to align the items along with the main-axis.
.justify-content {
display: flex;
justify-content: center;
flex-wrap: wrap;
height: 500px;
width: 500px;
background: lightgray;
padding: 5px;
}
.box {
background: tomato;
width: 100px;
height: 100px;
margin: 10px;
}
<div class="justify-content">
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
</div>
Align-content
align-content
is used to align the items inside the flexbox along the cross-axis. Note that it applies to Multi-line containers
.align-content {
display: flex;
align-content: center;
flex-wrap: wrap;
height: 500px;
width: 500px;
background: lightgray;
padding: 5px;
}
.box {
background: tomato;
width: 100px;
height: 100px;
margin: 10px;
}
<div class="align-content">
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
</div>
Align-items
align-items
has the same functionality as align-content
but the difference is that it works to center every single-line container instead of centering the whole container. Check that in the snippet, the whole container is divided into 250 pixels height each and centered within, while in align-content
it is centered within 500 pixels height of the row.
.align-items {
display: flex;
align-items: center;
flex-wrap: wrap;
height: 500px;
width: 500px;
background: lightgray;
padding: 5px;
}
.box {
background: tomato;
width: 100px;
height: 100px;
margin: 10px;
}
<div class="align-items">
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
</div>