This problem is NP-Hard, with a reduction from Knapsack-Problem.
Short intuitive "proof": The idea is, create a vertex for each item - you can either "take it" or "not take it" by choosing the vertex with the cost or the "free" vertex.
Sketch:
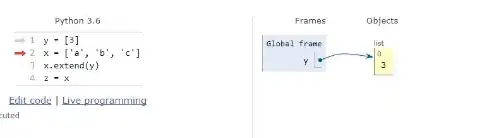
In the above you can see, from the Knapsack Problem, create a graph, for each item, you can choose to take it - and pay the cost and gain the "value", or ignore it.
More formally:
Given an instance of knapsack with weights=w1,w2,w3,...cn
and cost=c1,c2,..,cn
, with some maximal weight W
, create a graph G=(V,E)
with
V= { V_i,U_i,W_i | i=0,...n }
E= { (W_i,V_i+1,U_i+1 | i=0,...,n-1} U {(V_i,W_i+1), (U_i,W_i+1) | i=0,...,n-1 }
value(W_i,V_i+1) = c_i+1
money(W_i,V_i+1) = w_i+1
value(W_i,U_i+1) = 0
money(W_i,U_i+1) = 0
money(V_i,W_i+1) = cost(V_i,W_i+1) = money(U_i,W_i+1) = cost(U_i,W_i+1) = 0
The solution to this problem that uses at most W money, will be also the solution for knapsack with maximal capacity of W.
A possible pseudo polynomial solution could be (using Dynamic Programming technique):
D(start,0) = 0
D(v,x) = infinity x < 0
D(v,x) = min { D(u,x-money(u,v)) + value(u,v) | for each edge (u,v) } U {infinity}
In the above D(v,x)
is the minimal distance needed to travel from the start node to v
, paying exactly x
money.
Note that it can be done because it's a DAG, so you can calculate the values from first to last according to the graph's topological sort.
When done, you need to search all values of x
from 0
to MAXIMAL_AMOUNT_OF_MONEY_ALLOWED
to find the minimal value of D(target,x)
, and that's the answer.
Finding the actual cost is done by retracing back your steps, as done in other Dynamic Programming solutions.
The above run time is O(|V|*MAX_MONEY + |E|)