You should add the RefWatcher to your fragment as well just like what is described on the project page: https://github.com/square/leakcanary
LeakCanary.install() returns a pre configured RefWatcher. It also installs an ActivityRefWatcher that automatically detects if an activity is leaking after Activity.onDestroy() has been called.
public class ExampleApplication extends Application {
public static RefWatcher getRefWatcher(Context context) {
ExampleApplication application = (ExampleApplication) context.getApplicationContext();
return application.refWatcher;
}
private RefWatcher refWatcher;
@Override public void onCreate() {
super.onCreate();
refWatcher = LeakCanary.install(this);
}
}
You could use the RefWatcher to watch for fragment leaks:
public abstract class BaseFragment extends Fragment {
@Override public void onDestroy() {
super.onDestroy();
RefWatcher refWatcher = ExampleApplication.getRefWatcher(getActivity());
refWatcher.watch(this);
}
}
Besides, if you want to get the heap dump when memory leak happened, just open the Android Device Monitor from Android Studio, and select the tab "File Explorer". In the directory /mnt/shell/emulated/0/Download/leakcanary/detected_leaks, you will find all the heap dump files.
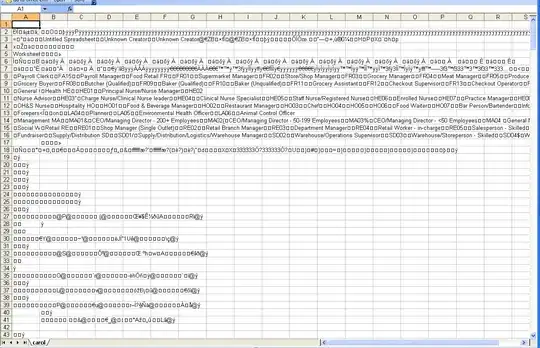