The error was a useful hint: a JointGrid organizes several subplots, you have to find the specific ax
to plot onto. :
import numpy as np
import pandas as pd
import seaborn as sns
from matplotlib.pyplot import show
sns.set_style('darkgrid')
# Generate a random correlated bivariate dataset
# https://stackoverflow.com/questions/16024677/generate-correlated-data-in-python-3-3
rs = np.random.RandomState(5)
mean = [0, 0]
cov = [[2, .1],
[.5, 3]] # make it asymmetric as a better test of x=y line
y = np.random.multivariate_normal(mean, cov, 500, tol=1e-4)
# Show the joint distribution using kernel density estimation
g = sns.jointplot(x=y[:,0], y=y[:,1],
kind="kde",
fill="true", height=5, space=0, levels=7)
# Draw a line of x=y
x0, x1 = g.ax_joint.get_xlim()
y0, y1 = g.ax_joint.get_ylim()
lims = [max(x0, y0), min(x1, y1)]
g.ax_joint.plot(lims, lims, '-r')
show()

I figured this out in an interpreter: dir(g)
, then g.plot?
, g.plot_joint?
-- those are plotting functions specific to the jointplot -- what else was there? -- dir(g.ax_joint)
; aha, there's set_ylim
, etc.
The diagonal line is the x=y line, but note that it isn't the 45deg diagonal of the pixels of the central plot. The seaborn jointplot
function always draws a square central plot. When the data is asymmetric the X and Y axes of the plot will change to fit into the square. The variable lims
holds the edges of the display in data coordinates.
There's a comment suggesting a diagonal plotted that will always be the diagonal of the display, but it isn't the line of equality of the data. Here are a few lines to add to test this and figure out which you want:
# This will always go from corner to corner of the displayed plot
g.ax_joint.plot([0,1], [0,1], ':y', transform=g.ax_joint.transAxes)
# This is the x=y line using transforms
g.ax_joint.plot(lims, lims, 'w', linestyle='dashdot', transform=g.ax_joint.transData)
# This is a simple way of checking which is x=y
g.ax_joint.scatter(x=[0, 2],y=[0,2], s=30,c= 'w',marker='o')
show()
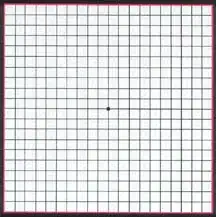