@Jordy van Eijk provided you with a viable solution but since you asked for an example I will provide you with my own implementation. Please note there are plenty of variations and other ways you may do this, and as your question seems to be quite broad I will only fill in the initial design.
My approach uses the MVVM design pattern:
A content presenter will bind to a view model and show the current data template. The data template will relate a view model to the user control. The user control contains your view, bindings to resize your selected item, and triggers to show/hide your extended display.
The Content Presenter (MainWindow.xaml):
<ContentPresenter Content="{Binding CurrentView}"/>
The Data Template (MainWindow.xaml):
<Window.Resources>
<DataTemplate DataType="{x:Type viewModel:UserControl1ViewModel}" >
<view:UserControl1/>
</DataTemplate>
</Window.Resources>
The User Control (UserControl1.xaml):
<UserControl.Resources>
<Style x:Key="ExtendedControl" TargetType="Button">
<Setter Property="Visibility" Value="Collapsed"/>
<Style.Triggers>
<DataTrigger Binding="{Binding IsVisible}" Value="True">
<Setter Property="Visibility" Value="Visible"/>
</DataTrigger>
</Style.Triggers>
</Style>
</UserControl.Resources>
<Grid HorizontalAlignment="Left" Background="Blue" Width="525">
<Button Content="Resize Control" VerticalAlignment="Top" HorizontalAlignment="Left" Width="{Binding Width}" Height="265" Command="{Binding ResizeCommand}" Margin="10,30,0,0"/>
<Button Content="Extended Control" Style="{StaticResource ExtendedControl}" Margin="383,32,25,258"/>
</Grid>
The User Control 1 View Model (UserControl1ViewModel.cs):
public class UserControl1ViewModel : ViewModelBase
{
public ICommand ResizeCommand
{
get
{
if (_resizeCommand == null) _resizeCommand = new RelayCommand(ResizeButton, () => true);
return _resizeCommand;
}
}
public bool IsVisible
{
get { return _isVisible; }
set
{
_isVisible = value;
RaisePropertyChanged("IsVisible");
}
}
public double Width
{
get { return _width; }
set
{
_width = value;
RaisePropertyChanged("Width");
}
}
private RelayCommand _resizeCommand;
private bool _isVisible;
private double _width;
public UserControl1ViewModel()
{
Width = 100.0;
IsVisible = false;
}
private void ResizeButton()
{
Width = Application.Current.MainWindow.ActualWidth * .65;
IsVisible = true;
}
}
Before Click:
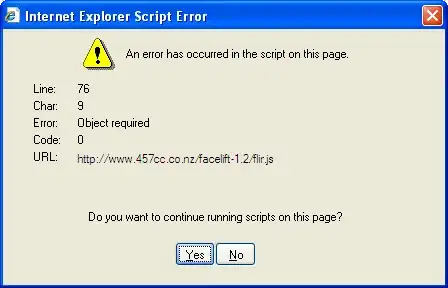
After Click:
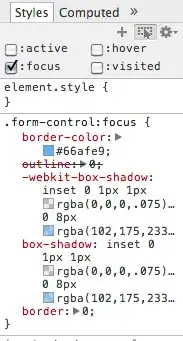
This outlines the main pieces you will need to create a base application like you are specifying. When the resize control is pressed, its width binding is changed to expand its size to 65% of the application main window and the visibility binding of the extended control button is changed to true. Id include pictures of the resize but my reputation doesn't allow it just yet. I hope you look into MVVM as a future architectural pattern as others have suggested and if you have any further question beyond my simple overview feel free to contact me. Good Luck!
Edit: the classes for the base view model, commands, and binding properties come from the MVVM Light library. You can import this into your project from visual studio using: Tools -> NuGet Package Manager -> Manage NuGet Packages For Solution -> search for "MVVM Light"
Edit 2
For an example related to your comment. We have a parent grid containing an editor view that is always at 70 percent of max window size and a binding for our extended control panel size:
View:
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="7*"/>
<ColumnDefinition Width="{Binding Width}"/>
</Grid.ColumnDefinitions>
<ContentPresenter Content="{Binding ViewManager.EditorControlView}" Grid.Column="0"/>
<ContentPresenter Content="{Binding ViewManager.ExtendedControlView}" Grid.Column="1"/>
</Grid>
Binding:
public string Width
{
get { return _width; }
set
{
_width = value;
RaisePropertyChanged("Width")
}
}
//set our extended control to the other 30 percent of the window size
Width = "3*";
To properly change this width adhering to MVVM standards, we need to use some form of communication between our view models and luckily that is another lesson you can pick up on from here. Just because iv used MVVM Light throughout this example id recommend searching google for "MVVM Light Messenger" as it provides a solid way of doing this communication. Using this you can just raise a change width to hide other window components in the parent view from anywhere in your application. :D