It took me a while to figure this one out, but it's actually quite simple.
Initially I created a custom class that extended the SearchView class, and used a onCreateInputConnection()
override, however I couldn't get it working that way.
I eventually got it working in a much more simple way, with just two added lines of code.
You just need to call search.getImeOptions()
to get the current configuration, and then "or" the result with EditorInfo.IME_FLAG_NO_EXTRACT_UI
with a call to setImeOptions()
:
Java:
search.setImeOptions(options|EditorInfo.IME_FLAG_NO_EXTRACT_UI);
Kotlin:
search.setImeOptions(search.imeOptions or EditorInfo.IME_FLAG_NO_EXTRACT_UI)
If you don't "or" with the existing options, then you don't get the "Search" completion button in the lower right, you just get a "Done" button instead.
Here is the full onCreateOptionsMenu()
override I used to test (I used a SearchView in the xml, but this solution should work for you even if you're not inflating your SearchView from xml):
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu_main, menu);
SearchManager manager = (SearchManager) getSystemService(Context.SEARCH_SERVICE);
SearchView search = (SearchView) menu.findItem(R.id.action_search).getActionView();
SearchableInfo si = manager.getSearchableInfo(getComponentName());
//Here is where the magic happens:
int options = search.getImeOptions();
search.setImeOptions(options|EditorInfo.IME_FLAG_NO_EXTRACT_UI);
//!!!!!!!!!!!
search.setSearchableInfo(si);
search.setOnQueryTextListener(new SearchView.OnQueryTextListener() {
@Override
public boolean onQueryTextSubmit(String query) {
return false;
}
@Override
public boolean onQueryTextChange(String query) {
return true;
}
});
return true;
}
Here is the xml I used for the SearchView in menu_main.xml:
<item android:id="@+id/action_search"
android:title="Search"
android:icon="@android:drawable/ic_menu_search"
app:showAsAction="always"
app:actionViewClass="android.support.v7.widget.SearchView"
/>
Result without the call to setImeOptions()
:
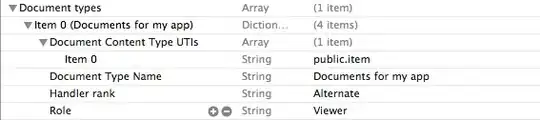
Result with the call to setImeOptions()
:
