So what you want to do, since it seems like UICollectionView works the same as UITableView, is make a subclass of UICollectionViewCell that contains a protocol to send actions, like pressing a button, to a view controller from a different view. In this case, a different view being the UICollectionViewCell.
Adding a Protocol to a UICollectionViewCell
Add a new Cocoa Touch Class called UICustomCollectionViewCell with subclass of UICollectionViewCell. And include the interface builder file
header file UICustomCollectionViewCell.h
@protocol UICustomCollectionViewCellDelegate;
@interface UICustomCollectionViewCell : UICollectionViewCell
@property ( nonatomic, retain) IBOutlet UIButton *button;
- (IBAction)pressButton:(id)sender;
@property ( assign) id< UICustomCollectionViewCellDelegate> delegate;
@end
@protocol UICustomCollectionViewCellDelegate <NSObject>
@optional
- (void)customCollectionViewCell:(UICustomCollectionViewCell *)cell pressedButton:(UIButton *)button;
@end
implementation file UICustomCollectionViewCell.m
@implementation UICustomCollectionViewCell
@synthesize delegate;
- (IBAction)pressButton:(id)sender {
if ([delegate respondsToSelector: @selector( customCollectionViewCell:pressedButton:)])
[delegate customCollectionViewCell: self pressedButton: sender];
}
@end
xib file UICustomCollectionViewCell.xib
make sure the connections from the UICustomCollectionViewCell are connected to the button from the Connections Inspector:
- button
- -pressButton:
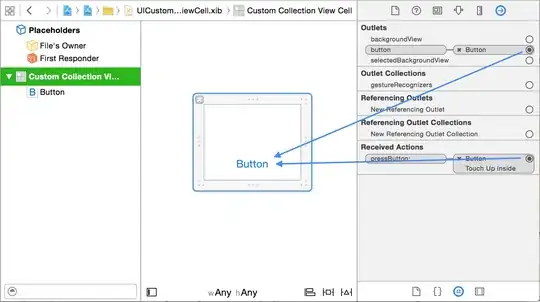
Finally, using this class in your project
Import the class as well as the delegate:
#import "UICustomCollectionViewCell.h"
@interface ViewController () < UICustomCollectionViewCellDelegate>
@end
In this following code, you will use the UICustomCollectionViewCell class instead of UICollectionViewCell:
UICustomCollectionViewCell *cell;
...
[cell setDelegate: self];
...
return cell;
And now the action, or method, that is called when the button is pressed:
- (void)customCollectionViewCell:(UICustomCollectionViewCell *)cell pressedButton:(UIButton *)button {
//action will be here when the button is pressed
}
If you want to find out what indexPath this cell was from:
[collectionView indexPathForCell: cell];