You need to understand the basics of Layout Managers before you can attempt anything with Swing.
Study the tutorial here https://docs.oracle.com/javase/tutorial/uiswing/layout/howLayoutWorks.html
If you are trying to construct a form with various Swing controls, never use absolute coordinates to position them. Resizing the window should dynamically reposition your controls according to the type of layout you want to use. If you are using your panel as a drawing canvas (which is what I think you are after), look at my example below.
Try to master a couple of fundamental layouts first such as BorderLayout and GridLayout. Once mastered you can use very few to achieve just about any kind of layout you desire by nesting them together. Also learn the basics of ScrollPane to make efficient use of screen real-estate
As for your original question, I created an example of how BorderLayout works and how to draw to a specific region on a panel. I also added some code so you can set your cursor differently depending on whether you are in the smaller region within a given panel.
Try this:
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Cursor;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Rectangle;
import java.awt.event.MouseEvent;
import java.awt.event.MouseMotionListener;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.SwingUtilities;
import javax.swing.WindowConstants;
public class FrameDemo extends JFrame {
private static final long serialVersionUID = 1L;
public FrameDemo() {
super("Frame Demo");
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
getContentPane().add(new CustomPanel(Color.RED), BorderLayout.NORTH);
getContentPane().add(new CustomPanel(Color.GREEN), BorderLayout.SOUTH);
getContentPane().add(new CustomPanel(Color.BLUE), BorderLayout.EAST);
getContentPane().add(new CustomPanel(Color.YELLOW), BorderLayout.WEST);
getContentPane().add(new JScrollPane(new CustomPanel(Color.BLACK)), BorderLayout.CENTER);
pack();
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
JFrame frame = new FrameDemo();
frame.setVisible(true);
}
});
}
}
class CustomPanel extends JPanel implements MouseMotionListener {
private static final long serialVersionUID = 1L;
private static final Dimension PANEL_SIZE = new Dimension(200, 100);
private static final int HAND_CURSOR_INDEX = 1;
private static final int DEFAULT_CURSOR_INDEX = 0;
private static final Cursor[] _cursors = new Cursor[] {
Cursor.getDefaultCursor(),
Cursor.getPredefinedCursor(Cursor.HAND_CURSOR)
};
// I want to place a JPanel with 300,200 size at 100,50 location in a JFrame
private static final Rectangle _rectangle = new Rectangle(50, 10, 40, 50);
public CustomPanel(Color color) {
setBackground(color);
addMouseMotionListener(this);
}
public Dimension getPreferredSize() {
return PANEL_SIZE;
}
public Dimension getMinimumSize() {
return PANEL_SIZE;
}
public Dimension getMaximumSize() {
return PANEL_SIZE;
}
protected void paintComponent(Graphics g) {
super.paintComponent(g);
g.setColor(Color.WHITE);
g.fillRect(
(int)_rectangle.getX(),
(int)_rectangle.getY(),
(int)_rectangle.getWidth(),
(int)_rectangle.getHeight());
}
@Override
public void mouseDragged(MouseEvent e) {
// TODO Auto-generated method stub
}
@Override
public void mouseMoved(MouseEvent e) {
int cursorIndex = _rectangle.contains(e.getPoint()) ?
HAND_CURSOR_INDEX :
DEFAULT_CURSOR_INDEX;
setCursor(_cursors[cursorIndex]);
}
}
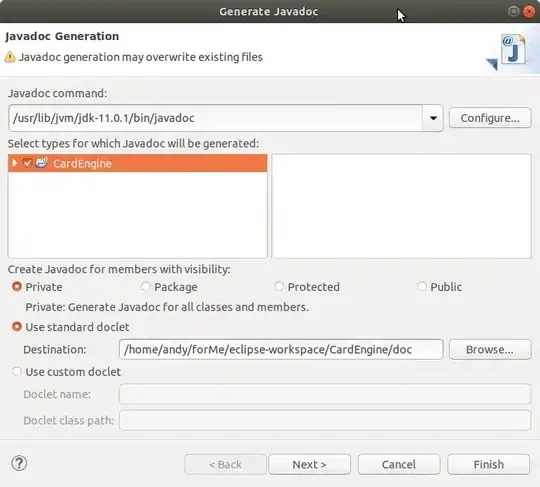