You code is correct
UIView *aView = [[UIView alloc] init];
aView.backgroundColor = [UIColor clearColor];
if([aView.backgroundColor isEqual:[UIColor clearColor]]) {
NSLog(@"Background is clear");
} else {
NSLog(@"Background is not clear");
}
Result:
2015-07-22 15:22:57.430 APP[1568:24173] Background is clear
UPDATE: case of default color from Interface builder
From : UIView Class Reference
@property(nonatomic, copy) UIColor *backgroundColor
Discussion
Changes to this property can be animated. The default value is nil,
which results in a transparent background color.
if you set color to be default from interface builder backgroundColor
property will be nil so the comparison to [UIColor clearColor]
will be false.
you can update you code the handle this case:
if([self.aView.backgroundColor isEqual:[UIColor clearColor]] || self.aView.backgroundColor == nil) {
NSLog(@"Background is clear");
} else {
NSLog(@"Background is not clear");
}
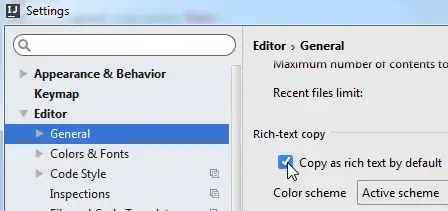
UPDATE: case of clear color from Interface builder
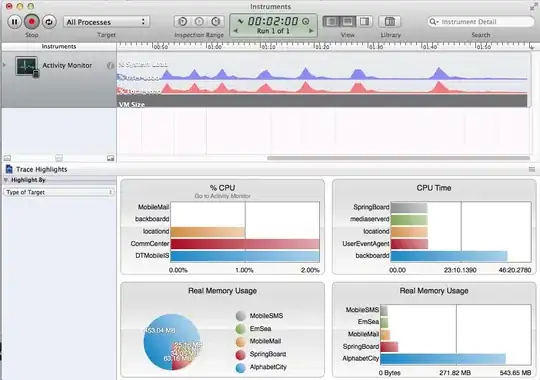
You can test the alpha value :
CGFloat bgColorAlpha = CGColorGetAlpha(self.aView.backgroundColor.CGColor);
if (bgColorAlpha == 0.0){
NSLog(@"clear ");
}else{
NSLog(@"not clear ");
}
2015-07-22 16:56:23.290 APP[1922:38283] clear
More information:
How to compare UIColors
Comparing colors in Objective-C
UIColor comparison