There are a couple approaches, as mwaskom tactfully pointed out. You can pass arguments to the joint plot, but setting color
there affects the whole scatterplot:
import numpy as np, pandas as pd; np.random.seed(0)
import seaborn as sns#; sns.set(style="white", color_codes=True)
tips = sns.load_dataset("tips")
g = sns.jointplot(x="total_bill", y="tip", data=tips, kind='reg',
joint_kws={'color':'green'}) # Scatter and regression all green
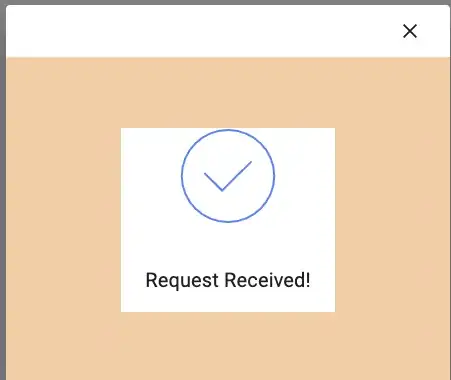
Or pass a dictionary of line-plotting keywords through that dictionary of scatterplot keywords. I read seaborn/linearmodels.py
to figure out where to do this, which was entertaining and informative in itself. Dict in dict:
g = sns.jointplot(x="total_bill", y="tip", data=tips, kind='reg',
joint_kws={'line_kws':{'color':'cyan'}}) # Only regression cyan
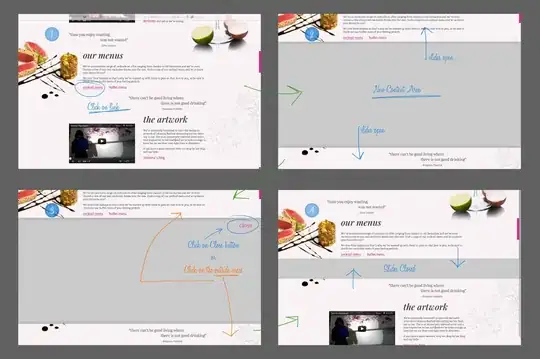
Or you can access the line after it's been plotted and change it directly. This depends on the regression line being the first line plotted, so could break with seaborn updates. It's also aesthetically/pedagogically different, as you don't recolor the uncertainty spread. It is a good way to get familiar with what the JointGrid
object is and how else you might interact with it. (And maybe there are properties you can't set with the function call arguments, although I can't think of any.)
g = sns.jointplot(x="total_bill", y="tip", data=tips, kind='reg')
regline = g.ax_joint.get_lines()[0]
regline.set_color('red')
regline.set_zorder(5)
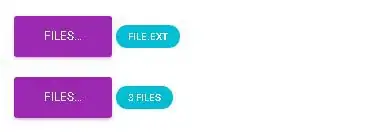